封装C++ modbus tcp客户端和服务端
时间: 2023-08-04 07:07:10 浏览: 86
这是一个基于 Boost 库封装的 C++ Modbus TCP 客户端和服务端的示例代码:
```cpp
#include <iostream>
#include <string>
#include <boost/asio.hpp>
#include <boost/asio/ip/tcp.hpp>
#include <boost/array.hpp>
#include <boost/thread.hpp>
using namespace std;
using namespace boost::asio;
using namespace boost::asio::ip;
// Modbus TCP 帧结构体
#pragma pack(push, 1)
struct ModbusTCPFrame {
uint16_t transaction_id;
uint16_t protocol_id;
uint16_t length;
uint8_t unit_id;
uint8_t function_code;
uint16_t start_address;
uint16_t quantity;
};
#pragma pack(pop)
// Modbus TCP 客户端类
class ModbusTCPClient {
public:
ModbusTCPClient(string ip_address, uint16_t port) :
m_ip_address(ip_address), m_port(port), m_socket(m_io_service) { }
// 连接到 Modbus TCP 服务器
bool connect() {
try {
m_socket.connect(tcp::endpoint(address::from_string(m_ip_address), m_port));
}
catch (boost::system::system_error& e) {
cerr << "Error: " << e.what() << endl;
return false;
}
return true;
}
// 发送读取线圈状态请求
bool read_coils(uint16_t start_address, uint16_t quantity, boost::array<uint8_t, 256>& response) {
ModbusTCPFrame frame = { 0 };
frame.transaction_id = 1;
frame.protocol_id = 0;
frame.length = 6;
frame.unit_id = 1;
frame.function_code = 0x01;
frame.start_address = htons(start_address);
frame.quantity = htons(quantity);
boost::system::error_code ec;
m_socket.write_some(buffer(&frame, 6), ec);
if (ec) {
cerr << "Error: " << ec.message() << endl;
return false;
}
size_t len = m_socket.read_some(buffer(response), ec);
if (ec) {
cerr << "Error: " << ec.message() << endl;
return false;
}
return true;
}
private:
string m_ip_address;
uint16_t m_port;
io_service m_io_service;
tcp::socket m_socket;
};
// Modbus TCP 服务端类
class ModbusTCPServer {
public:
ModbusTCPServer(uint16_t port) :
m_port(port), m_acceptor(m_io_service, tcp::endpoint(tcp::v4(), m_port)) { }
// 启动 Modbus TCP 服务器
void start() {
boost::thread t(boost::bind(&io_service::run, &m_io_service));
while (true) {
tcp::socket socket(m_io_service);
m_acceptor.accept(socket);
boost::thread(boost::bind(&ModbusTCPServer::handle_client, this, socket));
}
}
private:
void handle_client(tcp::socket& socket) {
boost::system::error_code ec;
while (true) {
boost::array<uint8_t, 256> request = { 0 };
size_t len = socket.read_some(buffer(request), ec);
if (ec) {
cerr << "Error: " << ec.message() << endl;
break;
}
ModbusTCPFrame* frame = (ModbusTCPFrame*)request.data();
if (frame->function_code == 0x01) {
uint16_t start_address = ntohs(frame->start_address);
uint16_t quantity = ntohs(frame->quantity);
boost::array<uint8_t, 256> response = { 0 };
response[0] = frame->transaction_id >> 8;
response[1] = frame->transaction_id;
response[2] = frame->protocol_id >> 8;
response[3] = frame->protocol_id;
response[4] = (quantity + 7) / 8;
for (int i = 0; i < quantity; i++) {
response[5 + i / 8] |= (i % 8 == 0 ? 0x80 : 0) | (i % 8 == 1 ? 0x40 : 0) | (i % 8 == 2 ? 0x20 : 0) | (i % 8 == 3 ? 0x10 : 0) | (i % 8 == 4 ? 0x08 : 0) | (i % 8 == 5 ? 0x04 : 0) | (i % 8 == 6 ? 0x02 : 0) | (i % 8 == 7 ? 0x01 : 0);
}
socket.write_some(buffer(response, 5 + (quantity + 7) / 8), ec);
if (ec) {
cerr << "Error: " << ec.message() << endl;
break;
}
}
}
}
private:
uint16_t m_port;
io_service m_io_service;
tcp::acceptor m_acceptor;
};
int main() {
// 创建 Modbus TCP 客户端对象并连接到服务器
ModbusTCPClient client("127.0.0.1", 502);
if (!client.connect()) {
return -1;
}
// 发送读取线圈状态请求并输出结果
boost::array<uint8_t, 256> response = { 0 };
if (client.read_coils(0, 16, response)) {
for (int i = 0; i < 16; i++) {
cout << (response[5 + i / 8] & (1 << (i % 8)) ? "1" : "0");
}
cout << endl;
}
// 创建 Modbus TCP 服务端对象并启动服务器
ModbusTCPServer server(502);
server.start();
return 0;
}
```
该代码使用 Boost 库实现了 Modbus TCP 客户端和服务端。Modbus TCP 帧结构体使用了 pragma pack 指令进行字节对齐。ModbusTCPClient 类实现了连接到 Modbus TCP 服务器和发送读取线圈状态请求的功能。ModbusTCPServer 类实现了启动 Modbus TCP 服务器和处理客户端请求的功能。在 main 函数中先创建 Modbus TCP 客户端对象并连接到服务器,然后发送读取线圈状态请求并输出结果。接着创建 Modbus TCP 服务端对象并启动服务器,等待客户端连接并处理客户端请求。
相关推荐
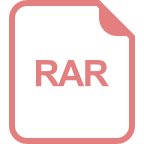
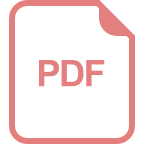














