用c语言写一个程序来统计给定txt文件中的字母、数字、空格、词语、特殊字符的数量
时间: 2024-01-29 13:02:15 浏览: 60
好的,我明白您的问题了。下面是一个用C语言编写的程序,可以统计给定txt文件中字母、数字、空格、词语、特殊字符的数量:
```
#include <stdio.h>
#include <ctype.h>
#define MAX_WORD_LEN 100
int main(int argc, char *argv[]) {
FILE *fp;
int c, prev_c = '\0';
int num_letters = 0, num_digits = 0, num_spaces = 0, num_words = 0, num_special_chars = 0;
char word[MAX_WORD_LEN + 1];
int word_len = 0;
if (argc != 2) {
fprintf(stderr, "Usage: %s filename\n", argv[0]);
return 1;
}
fp = fopen(argv[1], "r");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
while ((c = fgetc(fp)) != EOF) {
if (isalpha(c)) {
num_letters++;
} else if (isdigit(c)) {
num_digits++;
} else if (isspace(c)) {
num_spaces++;
if (prev_c != '\0' && !isspace(prev_c)) {
num_words++;
word[word_len] = '\0';
printf("Word: %s\n", word);
word_len = 0;
}
} else {
num_special_chars++;
}
if (!isspace(c)) {
if (word_len < MAX_WORD_LEN) {
word[word_len] = c;
word_len++;
}
}
prev_c = c;
}
if (prev_c != '\0' && !isspace(prev_c)) {
num_words++;
word[word_len] = '\0';
printf("Word: %s\n", word);
}
fclose(fp);
printf("Number of letters: %d\n", num_letters);
printf("Number of digits: %d\n", num_digits);
printf("Number of spaces: %d\n", num_spaces);
printf("Number of words: %d\n", num_words);
printf("Number of special characters: %d\n", num_special_chars);
return 0;
}
```
要运行程序,只需将txt文件作为参数传递给可执行文件。例如,如果可执行文件名为`count`,文件名为`test.txt`,则可以这样运行程序:
```
./count test.txt
```
程序将输出字母、数字、空格、词语和特殊字符的数量,并将每个词语打印出来。注意,这个程序假设每个词语之间由空格分隔,如果有其他分隔符,需要对代码进行修改。
阅读全文
相关推荐
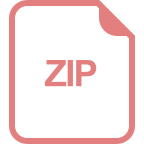















