用c++编写生命游戏,要求可以设置网格大小,模拟运行,直到用户触发停止信号,所需的以下功能 1run0):执行一次生成 2Display:显示当前状态 3lnit:初始化网格
时间: 2024-03-20 17:42:51 浏览: 17
好的,我会尽力回答你的问题。下面是用C++编写生命游戏的示例代码,实现了你所要求的功能:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
// 定义细胞的状态
enum CellState {
DEAD,
ALIVE
};
class LifeGame {
public:
// 构造函数
LifeGame(int row, int col): row_(row), col_(col), generation_(0) {
// 初始化矩阵
matrix_.resize(row_);
for (int i = 0; i < row_; ++i) {
matrix_[i].resize(col_);
for (int j = 0; j < col_; ++j) {
matrix_[i][j] = DEAD;
}
}
}
// 初始化矩阵
void Init() {
// 随机设置一些细胞的状态为ALIVE
srand((unsigned)time(nullptr));
for (int i = 0; i < row_; ++i) {
for (int j = 0; j < col_; ++j) {
matrix_[i][j] = (rand() % 2 == 0 ? DEAD : ALIVE);
}
}
}
// 执行一次生成
void Run() {
vector<vector<CellState>> new_matrix(row_, vector<CellState>(col_));
for (int i = 0; i < row_; ++i) {
for (int j = 0; j < col_; ++j) {
int count = CountAliveNeighbors(i, j);
if (matrix_[i][j] == ALIVE) {
if (count < 2 || count > 3) {
new_matrix[i][j] = DEAD;
} else {
new_matrix[i][j] = ALIVE;
}
} else {
if (count == 3) {
new_matrix[i][j] = ALIVE;
} else {
new_matrix[i][j] = DEAD;
}
}
}
}
matrix_ = new_matrix;
++generation_;
}
// 显示当前状态
void Display() {
cout << "Generation: " << generation_ << endl;
for (int i = 0; i < row_; ++i) {
for (int j = 0; j < col_; ++j) {
cout << (matrix_[i][j] == ALIVE ? "O " : ". ");
}
cout << endl;
}
cout << endl;
}
private:
// 统计(i, j)周围的存活细胞数
int CountAliveNeighbors(int i, int j) {
int count = 0;
for (int r = -1; r <= 1; ++r) {
for (int c = -1; c <= 1; ++c) {
int row = (i + r + row_) % row_;
int col = (j + c + col_) % col_;
if (matrix_[row][col] == ALIVE) {
++count;
}
}
}
if (matrix_[i][j] == ALIVE) {
--count;
}
return count;
}
int row_; // 矩阵的行数
int col_; // 矩阵的列数
int generation_; // 当前的代数
vector<vector<CellState>> matrix_; // 存储细胞状态的矩阵
};
int main() {
int row, col;
cout << "Input row and col: ";
cin >> row >> col;
LifeGame game(row, col);
game.Init();
while (true) {
cout << "1.Run 2.Display 3.Init 0.Exit" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
game.Run();
break;
case 2:
game.Display();
break;
case 3:
game.Init();
break;
case 0:
return 0;
default:
cout << "Invalid choice!" << endl;
break;
}
}
return 0;
}
```
这个程序实现了生命游戏的基本功能,包括初始化网格、执行一次生成、显示当前状态等。在程序中,我们使用一个二维数组来存储细胞的状态,用枚举类型CellState来表示细胞的状态,用一个类LifeGame来封装生命游戏的逻辑。在主函数中,我们通过不断地接收用户输入来执行不同的操作,直到用户选择退出程序为止。
相关推荐
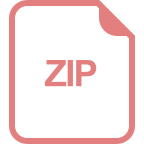
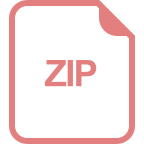














