background = cv2.cvtColor(background, cv2.COLOR_BGR2RGB)
时间: 2023-10-27 17:30:50 浏览: 36
This code converts the background image from BGR color space to RGB color space using the cv2.cvtColor function from the OpenCV library.
BGR (Blue, Green, Red) is the default color space used by OpenCV, while RGB (Red, Green, Blue) is the more common color space used in digital imaging.
Converting the image to RGB allows it to be displayed more accurately on devices that use RGB color space, such as computer monitors and mobile devices.
相关问题
以下代码是什么意思,请逐行解释:import tkinter as tk from tkinter import * import cv2 from PIL import Image, ImageTk import os import numpy as np global last_frame1 # creating global variable last_frame1 = np.zeros((480, 640, 3), dtype=np.uint8) global last_frame2 # creating global variable last_frame2 = np.zeros((480, 640, 3), dtype=np.uint8) global cap1 global cap2 cap1 = cv2.VideoCapture("./movie/video_1.mp4") cap2 = cv2.VideoCapture("./movie/video_1_sol.mp4") def show_vid(): if not cap1.isOpened(): print("cant open the camera1") flag1, frame1 = cap1.read() frame1 = cv2.resize(frame1, (600, 500)) if flag1 is None: print("Major error!") elif flag1: global last_frame1 last_frame1 = frame1.copy() pic = cv2.cvtColor(last_frame1, cv2.COLOR_BGR2RGB) img = Image.fromarray(pic) imgtk = ImageTk.PhotoImage(image=img) lmain.imgtk = imgtk lmain.configure(image=imgtk) lmain.after(10, show_vid) def show_vid2(): if not cap2.isOpened(): print("cant open the camera2") flag2, frame2 = cap2.read() frame2 = cv2.resize(frame2, (600, 500)) if flag2 is None: print("Major error2!") elif flag2: global last_frame2 last_frame2 = frame2.copy() pic2 = cv2.cvtColor(last_frame2, cv2.COLOR_BGR2RGB) img2 = Image.fromarray(pic2) img2tk = ImageTk.PhotoImage(image=img2) lmain2.img2tk = img2tk lmain2.configure(image=img2tk) lmain2.after(10, show_vid2) if __name__ == '__main__': root = tk.Tk() # img = ImageTk.PhotoImage(Image.open("logo.png")) heading = Label(root, text="Lane-Line Detection") # heading.configure(background='#CDCDCD',foreground='#364156') heading.pack() heading2 = Label(root, text="Lane-Line Detection", pady=20, font=('arial', 45, 'bold')) heading2.configure(foreground='#364156') heading2.pack() lmain = tk.Label(master=root) lmain2 = tk.Label(master=root) lmain.pack(side=LEFT) lmain2.pack(side=RIGHT) root.title("Lane-line detection") root.geometry("1250x900+100+10") exitbutton = Button(root, text='Quit', fg="red", command=root.destroy).pack(side=BOTTOM, ) show_vid() show_vid2() root.mainloop() cap.release()
这段代码是一个简单的图形用户界面(GUI),用于显示两个视频的帧。下面是每行代码的解释:
1. `import tkinter as tk`:导入 tkinter 库并将其命名为 tk,用于创建 GUI。
2. `from tkinter import *`:从 tkinter 库中导入所有的类和函数。
3. `import cv2`:导入 OpenCV 库,用于处理图像和视频。
4. `from PIL import Image, ImageTk`:导入 PIL 库,用于处理图像。
5. `import os`:导入 os 库,用于读取文件路径。
6. `import numpy as np`:导入 numpy 库,用于处理数组。
7. `global last_frame1`:声明一个全局变量 last_frame1,用于存储第一个视频的最后一帧。
8. `last_frame1 = np.zeros((480, 640, 3), dtype=np.uint8)`:初始化 last_frame1 变量为一个 480x640x3 的零数组。
9. `global last_frame2`:声明一个全局变量 last_frame2,用于存储第二个视频的最后一帧。
10. `last_frame2 = np.zeros((480, 640, 3), dtype=np.uint8)`:初始化 last_frame2 变量为一个 480x640x3 的零数组。
11. `global cap1`:声明一个全局变量 cap1,用于打开第一个视频文件。
12. `global cap2`:声明一个全局变量 cap2,用于打开第二个视频文件。
13. `cap1 = cv2.VideoCapture("./movie/video_1.mp4")`:打开第一个视频文件。
14. `cap2 = cv2.VideoCapture("./movie/video_1_sol.mp4")`:打开第二个视频文件。
15. `def show_vid():`:定义一个名为 show_vid 的函数,用于显示第一个视频的帧。
16. `if not cap1.isOpened():`:如果第一个视频文件无法打开,则输出错误信息。
17. `flag1, frame1 = cap1.read()`:读取第一个视频的一帧。
18. `frame1 = cv2.resize(frame1, (600, 500))`:将第一个视频的帧调整为 600x500 大小。
19. `if flag1 is None:`:如果读取的帧为 None,则输出错误信息。
20. `elif flag1:`:如果读取的帧存在,则执行以下操作。
21. `global last_frame1`:声明 last_frame1 是全局变量。
22. `last_frame1 = frame1.copy()`:将当前帧保存到 last_frame1 变量中。
23. `pic = cv2.cvtColor(last_frame1, cv2.COLOR_BGR2RGB)`:将 BGR 格式的图像转换为 RGB 格式。
24. `img = Image.fromarray(pic)`:将 ndarray 对象转换为 Image 对象。
25. `imgtk = ImageTk.PhotoImage(image=img)`:将 Image 对象转换为 ImageTk 对象。
26. `lmain.imgtk = imgtk`:将 imgtk 对象保存到 lmain 对象的 imgtk 属性中。
27. `lmain.configure(image=imgtk)`:将 imgtk 对象显示在 lmain 对象上。
28. `lmain.after(10, show_vid)`:每隔 10 毫秒调用 show_vid 函数一次。
29. `def show_vid2():`:定义一个名为 show_vid2 的函数,用于显示第二个视频的帧。
30. `if not cap2.isOpened():`:如果第二个视频文件无法打开,则输出错误信息。
31. `flag2, frame2 = cap2.read()`:读取第二个视频的一帧。
32. `frame2 = cv2.resize(frame2, (600, 500))`:将第二个视频的帧调整为 600x500 大小。
33. `if flag2 is None:`:如果读取的帧为 None,则输出错误信息。
34. `elif flag2:`:如果读取的帧存在,则执行以下操作。
35. `global last_frame2`:声明 last_frame2 是全局变量。
36. `last_frame2 = frame2.copy()`:将当前帧保存到 last_frame2 变量中。
37. `pic2 = cv2.cvtColor(last_frame2, cv2.COLOR_BGR2RGB)`:将 BGR 格式的图像转换为 RGB 格式。
38. `img2 = Image.fromarray(pic2)`:将 ndarray 对象转换为 Image 对象。
39. `img2tk = ImageTk.PhotoImage(image=img2)`:将 Image 对象转换为 ImageTk 对象。
40. `lmain2.img2tk = img2tk`:将 img2tk 对象保存到 lmain2 对象的 img2tk 属性中。
41. `lmain2.configure(image=img2tk)`:将 img2tk 对象显示在 lmain2 对象上。
42. `lmain2.after(10, show_vid2)`:每隔 10 毫秒调用 show_vid2 函数一次。
43. `if __name__ == '__main__':`:如果该模块是作为主程序运行,则执行以下操作。
44. `root = tk.Tk()`:创建一个名为 root 的 Tk 对象,用于创建 GUI 窗口。
45. `heading = Label(root, text="Lane-Line Detection")`:创建一个名为 heading 的 Label 对象,用于显示文本。
46. `heading.pack()`:将 heading 对象显示在窗口中。
47. `heading2 = Label(root, text="Lane-Line Detection", pady=20, font=('arial', 45, 'bold'))`:创建一个名为 heading2 的 Label 对象,用于显示文本。
48. `heading2.configure(foreground='#364156')`:设置 heading2 对象的前景色为 '#364156'。
49. `heading2.pack()`:将 heading2 对象显示在窗口中。
50. `lmain = tk.Label(master=root)`:创建一个名为 lmain 的 Label 对象。
51. `lmain2 = tk.Label(master=root)`:创建一个名为 lmain2 的 Label 对象。
52. `lmain.pack(side=LEFT)`:将 lmain 对象显示在窗口的左侧。
53. `lmain2.pack(side=RIGHT)`:将 lmain2 对象显示在窗口的右侧。
54. `root.title("Lane-line detection")`:设置窗口的标题为 "Lane-line detection"。
55. `root.geometry("1250x900+100+10")`:设置窗口的大小和位置。
56. `exitbutton = Button(root, text='Quit', fg="red", command=root.destroy).pack(side=BOTTOM, )`:创建一个名为 exitbutton 的 Button 对象,用于退出程序。
57. `show_vid()`:调用 show_vid 函数,显示第一个视频的帧。
58. `show_vid2()`:调用 show_vid2 函数,显示第二个视频的帧。
59. `root.mainloop()`:进入 GUI 的事件循环。
60. `cap.release()`:释放视频文件。
import cv2 import numpy as np from PIL import Image, ImageDraw, ImageFont # 视频分辨率 VIDEO_WIDTH = 1920 VIDEO_HEIGHT = 1080 # 文本属性 FONT_SIZE = int(VIDEO_HEIGHT * 0.75) FONT_COLOR = (255, 255, 255) # 背景属性 BACKGROUND_COLOR = (255,0,0,255) # 文本滚动速度 SCROLL_SPEED = int(VIDEO_WIDTH / 100) # 跑马灯文本 MARQUEE_TEXT = "Hello World! This is a marquee text." # 创建视频输出对象 fourcc = cv2.VideoWriter_fourcc(*'mp4v') video_writer = cv2.VideoWriter("E:/Template/word/marquee.mp4", fourcc, 25, (VIDEO_WIDTH, VIDEO_HEIGHT)) # 创建画布 canvas = Image.new('RGB', (VIDEO_WIDTH, VIDEO_HEIGHT), BACKGROUND_COLOR) draw = ImageDraw.Draw(canvas) # 加载字体 font = ImageFont.truetype("arial.ttf", FONT_SIZE) # 计算文本宽度 text_width, text_height = draw.textsize(MARQUEE_TEXT, font=font) # 文本起始位置 x = VIDEO_WIDTH # 循环生成帧并写入视频文件 while x > -text_width: # 添加文本 draw.text((x, (VIDEO_HEIGHT - text_height) / 2), MARQUEE_TEXT, font=font, fill=FONT_COLOR) # 转换图像格式 frame = np.array(canvas) frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR) # 写入视频文件 video_writer.write(frame) # 清空画布 draw.rectangle((0, 0, VIDEO_WIDTH, VIDEO_HEIGHT), fill=BACKGROUND_COLOR) # 更新文本位置 x -= SCROLL_SPEED # 释放资源 video_writer.release() 优化代码,加入导出进度条百分比
import cv2
import numpy as np
from PIL import Image, ImageDraw, ImageFont
# 视频分辨率
VIDEO_WIDTH = 1920
VIDEO_HEIGHT = 1080
# 文本属性
FONT_SIZE = int(VIDEO_HEIGHT * 0.75)
FONT_COLOR = (255, 255, 255)
# 背景属性
BACKGROUND_COLOR = (255,0,0,255)
# 文本滚动速度
SCROLL_SPEED = int(VIDEO_WIDTH / 100)
# 跑马灯文本
MARQUEE_TEXT = "Hello World! This is a marquee text."
# 创建视频输出对象
fourcc = cv2.VideoWriter_fourcc(*'mp4v')
video_writer = cv2.VideoWriter("E:/Template/word/marquee.mp4", fourcc, 25, (VIDEO_WIDTH, VIDEO_HEIGHT))
# 创建画布
canvas = Image.new('RGB', (VIDEO_WIDTH, VIDEO_HEIGHT), BACKGROUND_COLOR)
draw = ImageDraw.Draw(canvas)
# 加载字体
font = ImageFont.truetype("arial.ttf", FONT_SIZE)
# 计算文本宽度
text_width, text_height = draw.textsize(MARQUEE_TEXT, font=font)
# 文本起始位置
x = VIDEO_WIDTH
# 循环生成帧并写入视频文件
while x > -text_width:
# 添加文本
draw.text((x, (VIDEO_HEIGHT - text_height) / 2), MARQUEE_TEXT, font=font, fill=FONT_COLOR)
# 转换图像格式
frame = np.array(canvas)
frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR)
# 写入视频文件
video_writer.write(frame)
# 清空画布
draw.rectangle((0, 0, VIDEO_WIDTH, VIDEO_HEIGHT), fill=BACKGROUND_COLOR)
# 更新文本位置
x -= SCROLL_SPEED
# 导出进度条百分比
progress = int((x / VIDEO_WIDTH) * 100)
print("Exporting video: {}%".format(progress), end='\r')
# 释放资源
video_writer.release()
相关推荐
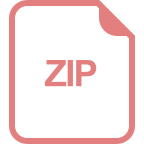
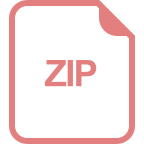












