在python中用字典表示多项式
时间: 2023-11-16 22:07:04 浏览: 104
在 Python 中,我们可以使用字典来表示多项式。字典中的键表示该项的指数,值表示该项的系数。
例如,多项式 2x^3 + 3x^2 - x + 5 可以表示为以下字典:
```python
poly = {3: 2, 2: 3, 1: -1, 0: 5}
```
其中,键 3 表示 x 的三次方项,值 2 表示该项的系数 2;键 2 表示 x 的二次方项,值 3 表示该项的系数 3,以此类推。
可以使用以下代码来实现多项式的加法:
```python
def poly_add(p1, p2):
res = {}
for exp in p1:
if exp in p2:
res[exp] = p1[exp] + p2[exp]
else:
res[exp] = p1[exp]
for exp in p2:
if exp not in res:
res[exp] = p2[exp]
return res
```
其中,`p1` 和 `p2` 分别表示两个多项式,返回值为它们的和。
相关问题
python中字典表示多项式
在Python中,可以使用字典来表示多项式。其中,字典的键表示多项式中的指数,字典的值表示多项式中该指数对应的系数。
例如,多项式 2x^3 + 4x^2 - 3x + 1 可以表示为如下的字典形式:
```
poly = {3: 2, 2: 4, 1: -3, 0: 1}
```
其中,键为指数,值为对应的系数。可以使用字典的方法来进行多项式的运算,例如:
```
# 多项式加法
def poly_add(p1, p2):
res = {}
for k in p1:
res[k] = p1.get(k, 0) + p2.get(k, 0)
for k in p2:
if k not in res:
res[k] = p2[k]
return res
# 多项式乘法
def poly_mul(p1, p2):
res = {}
for k1 in p1:
for k2 in p2:
k = k1 + k2
res[k] = res.get(k, 0) + p1[k1] * p2[k2]
return res
```
通过这种方式,可以方便地表示和操作多项式。
python链表多项式加多项式
以下是Python实现的链表多项式加多项式的示例代码:
```python
class Node:
def __init__(self, coeff, exp, next=None):
self.coeff = coeff
self.exp = exp
self.next = next
class Polynomial:
def __init__(self, head=None):
self.head = head
def insert(self, coeff, exp):
if self.head is None:
self.head = Node(coeff, exp)
else:
curr = self.head
while curr.next is not None:
curr = curr.next
curr.next = Node(coeff, exp)
def add(self, poly2):
result = Polynomial()
curr1 = self.head
curr2 = poly2.head
while curr1 is not None and curr2 is not None:
if curr1.exp > curr2.exp:
result.insert(curr1.coeff, curr1.exp)
curr1 = curr1.next
elif curr1.exp < curr2.exp:
result.insert(curr2.coeff, curr2.exp)
curr2 = curr2.next
else:
result.insert(curr1.coeff + curr2.coeff, curr1.exp)
curr1 = curr1.next
curr2 = curr2.next
while curr1 is not None:
result.insert(curr1.coeff, curr1.exp)
curr1 = curr1.next
while curr2 is not None:
result.insert(curr2.coeff, curr2.exp)
curr2 = curr2.next
return result
def display(self):
curr = self.head
while curr is not None:
print(curr.coeff, "x^", curr.exp, end=" ")
curr = curr.next
print()
# 示例
p1 = Polynomial()
p1.insert(1, 2)
p1.insert(2, 1)
p1.insert(3, 0)
p2 = Polynomial()
p2.insert(2, 1)
p2.insert(1, 0)
p3 = p1.add(p2)
p1.display() # 输出 "1 x^ 2 2 x^ 1 3 x^ 0"
p2.display() # 输出 "2 x^ 1 1 x^ 0"
p3.display() # 输出 "1 x^ 2 4 x^ 1 4 x^ 0"
```
以上代码中,`Node`类表示链表节点,包含系数和指数两个属性,以及指向下一个节点的指针。`Polynomial`类表示多项式,包含一个链表作为成员变量,支持插入节点、多项式加法和显示多项式等操作。在多项式加法中,使用两个指针分别指向两个多项式的头节点,遍历链表将相同指数的项相加,并将结果插入到结果多项式中。最后,将剩余的项依次插入到结果多项式中。
阅读全文
相关推荐
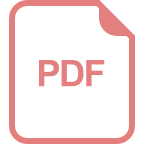
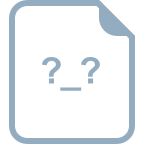
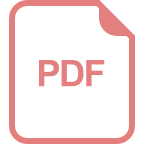













