construct an object
时间: 2024-03-13 10:45:19 浏览: 20
Sure, to construct an object we need to follow these steps:
1. Create a class for the object that defines its properties and methods.
2. Instantiate the class by creating an object of that class.
3. Set the initial values of the object's properties using the constructor.
4. Call the object's methods to perform actions or access its properties.
Here's an example of how to construct an object in Python:
```
# Define a class for a car
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer = 0
def drive(self, miles):
self.odometer += miles
# Instantiate a car object
my_car = Car("Toyota", "Corolla", 2021)
# Access and print the object's properties
print(my_car.make)
print(my_car.model)
print(my_car.year)
# Call the object's method to drive 100 miles
my_car.drive(100)
# Access and print the object's updated odometer property
print(my_car.odometer)
```
Output:
```
Toyota
Corolla
2021
100
```
相关推荐
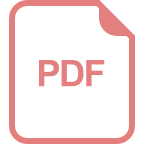
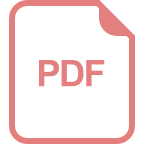
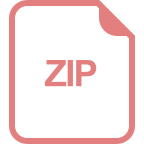














