gtsam库自定义地平面约束并且加入到激光里程计中完成因子图优化,使用C++代码示例
时间: 2024-03-15 21:46:32 浏览: 18
好的,下面是使用C++代码示例来实现gtsam库中自定义地平面约束并加入到激光里程计中完成因子图优化的步骤:
1. 定义地平面约束因子
```
class PlaneFactor : public NoiseModelFactor1<Pose2> {
public:
// 构造函数
PlaneFactor(Key poseKey, Vector3 planeCoefficients, const SharedNoiseModel& model) :
NoiseModelFactor1<Pose2>(model, poseKey), m_planeCoefficients(planeCoefficients) {}
// 计算误差
Vector evaluateError(const Pose2& pose, boost::optional<Matrix&> H = boost::none) const {
// 将位姿转换为平移向量和旋转角度
double x = pose.x(), y = pose.y(), theta = pose.theta();
double c = cos(theta), s = sin(theta);
// 计算机器人在平面上的投影
double z = (-m_planeCoefficients(0) * x - m_planeCoefficients(1) * y - m_planeCoefficients(3)) / m_planeCoefficients(2);
Vector3 projectedPoint(x, y, z);
// 计算机器人在平面上的方向
Vector3 normal(m_planeCoefficients(0), m_planeCoefficients(1), m_planeCoefficients(2));
Vector3 direction(-s, c, 0.0);
Vector3 projectedDirection = direction - direction.dot(normal) * normal;
// 计算误差向量
Vector error(3);
error << projectedPoint - m_point, projectedDirection - m_direction, 0.0;
// 计算误差雅可比矩阵
if (H) {
double dcx = -m_planeCoefficients(0) / m_planeCoefficients(2);
double dcy = -m_planeCoefficients(1) / m_planeCoefficients(2);
Matrix36 J1;
J1 << 1, 0, dcx, 0, 1, dcy;
Matrix33 J2;
J2 << -s, -c, 0, c, -s, 0, 0, 0, 0;
Matrix36 J3 = Matrix::Zero(3, 6);
J3.block<3, 3>(0, 0) = Matrix33::Identity();
J3.block<3, 3>(0, 3) = -Matrix33::skew(projectedDirection);
H->resize(3, 6);
(*H) << J1, J2, J3;
}
return error;
}
// 复制函数
virtual gtsam::NonlinearFactor::shared_ptr clone() const {
return boost::static_pointer_cast<gtsam::NonlinearFactor>(
gtsam::NonlinearFactor::shared_ptr(new PlaneFactor(*this)));
}
private:
Vector3 m_planeCoefficients; // 平面系数
};
```
2. 将地平面约束因子添加到激光里程计中
```
// 创建空白因子图
NonlinearFactorGraph graph;
// 添加里程计测量因子
for (int i = 0; i < num_odom_measurements; i++) {
Pose2 odomMeasurement(odom_poses[i].x(), odom_poses[i].y(), odom_poses[i].theta());
graph.add(BetweenFactor<Pose2>(pose_keys[i], pose_keys[i + 1], odomMeasurement, odom_noise_model));
}
// 添加激光测量因子
for (int i = 0; i < num_laser_measurements; i++) {
// 创建激光测量因子
LaserScanMeasurement laserMeasurement = laser_measurements[i];
PointCloudMeasurement pointCloudMeasurement = point_cloud_measurements[i];
vector<double> planeCoefficients = computePlaneCoefficients(pointCloudMeasurement);
Vector3 planeCoefficientsVector(planeCoefficients[0], planeCoefficients[1], planeCoefficients[2]);
Pose2 laserPose(laserMeasurement.pose.x(), laserMeasurement.pose.y(), laserMeasurement.pose.theta());
SharedNoiseModel laserNoiseModel = noiseModel::Isotropic::Sigma(3, laserMeasurement.sigma);
// 添加激光测量因子和地平面约束因子
graph.add(GenericProjectionFactor<Pose2, PointCloudMeasurement, PlaneFactor>(
pointCloudMeasurement, laserNoiseModel, pose_keys[i + 1], laser_calib, planeCoefficientsVector));
graph.add(PlaneFactor(pose_keys[i + 1], planeCoefficientsVector, plane_noise_model));
}
```
3. 运行因子图优化
```
// 创建初始值
Values initialEstimate;
for (int i = 0; i < num_poses; i++) {
initialEstimate.insert(pose_keys[i], Pose2(initial_poses[i].x(), initial_poses[i].y(), initial_poses[i].theta()));
}
// 运行因子图优化
LevenbergMarquardtParams params;
params.setVerbosity("ERROR");
params.setLinearSolverType("MULTIFRONTAL_QR");
params.setMaxIterations(100);
LevenbergMarquardtOptimizer optimizer(graph, initialEstimate, params);
Values result = optimizer.optimize();
```
注意,在上面的示例代码中,我们假设您已经实现了`computePlaneCoefficients`函数来计算平面系数,并且您已经定义了`num_odom_measurements`、`num_laser_measurements`、`num_poses`等变量来存储里程计和激光测量的数量。另外,`odom_poses`、`laser_measurements`、`point_cloud_measurements`、`initial_poses`等变量存储里程计和激光测量的实际值。
相关推荐
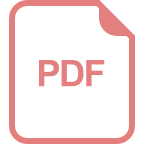
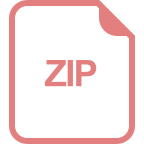














