java自主顺序栈实现 假设栈的输入序列为1、2、3、...、n,设计算法求出所有可能的出栈序列.并在命令行打印输出
时间: 2024-01-12 17:05:33 浏览: 23
以下是Java代码实现:
```java
import java.util.*;
public class StackPermutation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入栈的大小:");
int n = scanner.nextInt();
List<List<Integer>> permutations = getStackPermutations(n);
System.out.println("所有可能的出栈序列为:");
for (List<Integer> permutation : permutations) {
System.out.println(permutation);
}
}
public static List<List<Integer>> getStackPermutations(int n) {
List<List<Integer>> permutations = new ArrayList<>();
if (n == 0) {
permutations.add(new ArrayList<>());
return permutations;
}
List<Integer> stack = new ArrayList<>();
for (int i = 1; i <= n; i++) {
stack.add(i);
}
getStackPermutationsHelper(stack, new ArrayList<>(), new Stack<>(), permutations);
return permutations;
}
private static void getStackPermutationsHelper(List<Integer> stack, List<Integer> permutation, Stack<Integer> tempStack, List<List<Integer>> permutations) {
if (stack.isEmpty() && tempStack.isEmpty()) {
permutations.add(new ArrayList<>(permutation));
return;
}
if (!tempStack.isEmpty()) {
int temp = tempStack.pop();
permutation.add(temp);
getStackPermutationsHelper(stack, permutation, tempStack, permutations);
permutation.remove(permutation.size() - 1);
tempStack.push(temp);
}
if (!stack.isEmpty()) {
int num = stack.remove(0);
tempStack.push(num);
getStackPermutationsHelper(stack, permutation, tempStack, permutations);
stack.add(0, num);
tempStack.pop();
}
}
}
```
代码逻辑如下:
1. 首先根据输入的大小n创建一个初始栈,栈中从1到n依次排列;
2. 然后调用helper函数,该函数用于递归求解所有可能的出栈序列;
3. 在helper函数中,首先判断tempStack是否为空,若不为空,则将tempStack栈顶元素弹出,加入permutation序列中,然后递归调用helper函数,再从permutation序列中将该元素移除,将该元素重新压入tempStack中;
4. 接着判断stack是否为空,若不为空,则将stack栈顶元素取出,压入tempStack中,递归调用helper函数,再将该元素移回stack中,弹出tempStack栈顶元素。
在递归过程中,当stack和tempStack均为空时,则表示已经求出一种出栈序列,将其加入permutations序列中。最后返回所有可能的出栈序列。
相关推荐
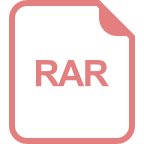
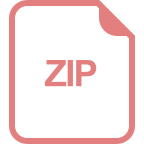














