#include <iostream>#include <stack>#include <string>using namespace std;template <typename T>class Stack {public: void push(T value) { s_.push(value); } void pop() { s_.pop(); } T top() { return s_.top(); } bool empty() { return s_.empty(); }private: stack<T> s_;};int main() { int num, base; cout << "请输入要转换的十进制数和目标进制数(2-16):" << endl; cin >> num >> base; Stack<char> s; while (num != 0) { int remainder = num % base; char c; if (remainder < 10) { c = remainder + '0'; } else { c = remainder - 10 + 'A'; } s.push(c); num /= base; } cout << "转换结果为:" << endl; while (!s.empty()) { cout << s.top(); s.pop(); } cout << endl; return 0;}这段代码中,为什么remainder的值小于10就让c加上'0',反之则让c减去10加上'A'
时间: 2024-02-29 13:56:04 浏览: 58
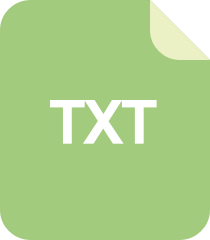
使用include <iostream>时一定要加using namespace std
这是因为在进制转换中,当余数小于10时,直接转换成字符即可,例如余数为3,则转换成字符'3'。而当余数大于等于10时,需要用字母表示,例如余数为10,则转换成字符'A',余数为11,则转换成字符'B',以此类推。因此在代码中,当余数小于10时,需要加上字符'0'来转换成对应的数字字符,当余数大于等于10时,需要减去10并加上字符'A'来转换成对应的字母字符。
阅读全文
相关推荐
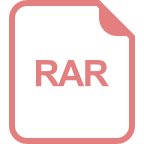
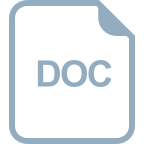








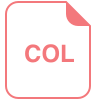
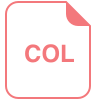
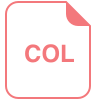
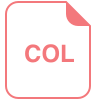
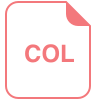
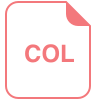
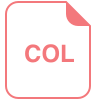
