c语言and和or的用法
时间: 2024-02-03 22:15:52 浏览: 29
在 C 语言中,`and` 和 `or` 是逻辑运算符,用于对表达式进行逻辑运算,返回结果为 `true` 或 `false`。
`and` 和 `or` 的作用与 `&&` 和 `||` 相同,只是 `and` 和 `or` 的优先级较低,因此需要用括号来明确运算顺序。例如:
```c
if (x > 0 and y < 10) {
// 如果 x 大于 0 并且 y 小于 10,执行这里的代码
}
if (a == 0 or b == 0) {
// 如果 a 等于 0 或者 b 等于 0,执行这里的代码
}
```
注意,在 C 语言中,`and` 和 `or` 是非短路运算符,即无论第一个操作数的值是什么,都会计算第二个操作数的值,因此可能会出现不必要的计算,导致程序效率降低。建议在实际开发中使用 `&&` 和 `||`。
相关问题
C语言里&&和||的区别
在C语言中,&&和||是逻辑运算符,用于进行逻辑与和逻辑或运算。
1. 逻辑与运算符(&&):
- 当两个操作数都为真(非零)时,逻辑与运算的结果为真(非零)。
- 如果其中一个操作数为假(零),则整个表达式的结果为假(零)。
- 逻辑与运算符具有短路特性,即如果第一个操作数为假(零),则不会计算第二个操作数。
2. 逻辑或运算符(||):
- 当两个操作数中至少有一个为真(非零)时,逻辑或运算的结果为真(非零)。
- 如果两个操作数都为假(零),则整个表达式的结果为假(零)。
- 逻辑或运算符也具有短路特性,即如果第一个操作数为真(非零),则不会计算第二个操作数。
下面是一个示例代码,演示了C语言中逻辑与(&&)和逻辑或(||)的用法:
```c
#include <stdio.h>
int main() {
int a = 5;
int b = 10;
int c = 0;
// 逻辑与运算
if (a > 0 && b > 0) {
printf("Both a and b are positive.\n");
}
// 逻辑或运算
if (a > 0 || c > 0) {
printf("Either a or c is positive.\n");
}
return 0;
}
```
输出结果:
```
Both a and b are positive.
Either a or c is positive.
```
mybatisplus使用or函数
在MyBatis Plus中,使用or函数可以在查询条件中实现or逻辑操作。例如,可以使用LambdaQueryWrapper类的or方法来将多个查询条件进行or连接。下面是一个示例:
```
customerMapper.selectList(new LambdaQueryWrapper<Customer>()
.eq(Customer::getTaxpayerIdentificationNumber,"912102113000000000")
.and(qw -> qw.eq(Customer::getParentId,111).or().eq(Customer::getContactNumber,"88"))
.eq(Customer::getName, "啦啦啦")
.or()
.ne(Customer::getNumber, 1)
.eq(Customer::getCompanyType,1));
```
在这个示例中,使用了LambdaQueryWrapper类的eq方法来添加一个等于条件,然后使用and方法来添加一个and逻辑操作,接着使用or方法来添加一个or逻辑操作,最后使用eq方法添加另一个等于条件,并且使用or方法来添加一个or逻辑操作。这样就能够实现or逻辑操作。
引用提供的代码示例展示了如何使用or函数和LambdaQueryWrapper类来构建含有or逻辑操作的查询条件。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [MATLAB中isequal函数转化为C语言,有项目算法使用matlab中isequal函数进行运算,这里需要将转化为C语言](https://download.csdn.net/download/li171049/88279963)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [MybatisPlus 中and和or的使用,附有对应的sql语句,有助于理解。](https://blog.csdn.net/lucky_she_niu/article/details/126020429)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
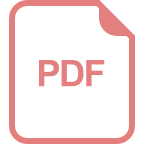
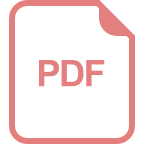
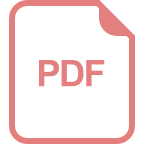












