area = (boxes[:, 3] - boxes[:, 1]) * (boxes[:, 2] - boxes[:, 0]) IndexError: too many indices for tensor of dimension
时间: 2024-05-27 21:11:37 浏览: 23
2
This error message is indicating that there are too many indices being used to access a tensor of dimension 2.
In this specific case, it seems that the variable `boxes` is a 2D tensor (i.e. a matrix) where each row represents a bounding box. The error message is telling us that the expression `boxes[:, 3]` is trying to access the fourth column of the matrix, which doesn't exist.
To fix this error, we need to make sure that the matrix `boxes` has at least 4 columns, or adjust the indices used to access the matrix accordingly.
相关问题
area = (boxes[:, 3] - boxes[:, 1]) * (boxes[:, 2] - boxes[:, 0]) IndexError: too many indices for tensor of dimension 1
This error occurs when trying to access multiple columns of a tensor with a single index.
For example, if we have a tensor of size (10, 4) where each row represents a box with 4 values (x1, y1, x2, y2), and we want to compute the area of each box, we need to access two columns of the tensor for each box.
If we try to access multiple columns with a single index, we get the "IndexError: too many indices for tensor of dimension 1" error.
To fix this error, we need to make sure that we are accessing the correct columns of the tensor. In the case of computing the area of boxes, we need to access the columns for x1, y1, x2, and y2 separately, like this:
```
area = (boxes[:, 2] - boxes[:, 0]) * (boxes[:, 3] - boxes[:, 1])
```
This will compute the area of each box and return a tensor of size (10,).
def box_iou(boxes1, boxes2): area1 = box_area(boxes1) area2 = box_area(boxes2) lt = torch.max(boxes1[:, None, :2], boxes2[:, :2]) # [N,M,2] rb = torch.min(boxes1[:, None, 2:], boxes2[:, 2:]) # [N,M,2] wh = (rb - lt).clamp(min=0) # [N,M,2] inter = wh[:, :, 0] * wh[:, :, 1] # [N,M] union = area1[:, None] + area2 - inter iou = inter / union return iou, union
这段代码是一个计算两个框(boxes)之间的IoU(Intersection over Union)的函数。IoU是用来衡量两个框的重叠程度的指标,范围在0到1之间,值越接近1表示两个框的重叠程度越高。
代码中的`boxes1`和`boxes2`分别表示两组框的坐标信息。首先,通过调用`box_area`函数分别计算了两组框的面积,分别存储在`area1`和`area2`中。
然后,通过使用`torch.max`函数计算了两组框左上角坐标的最大值,再使用`torch.min`函数计算了两组框右下角坐标的最小值。这样就得到了两组框的左上角和右下角坐标的交集区域,存储在`lt`和`rb`中。
接下来,通过计算交集区域的宽度和高度,即`(rb - lt).clamp(min=0)`,得到了交集区域的宽度和高度,存储在`wh`中。
然后,通过计算交集区域的面积,即`wh[:, :, 0] * wh[:, :, 1]`,得到了交集区域的面积,存储在`inter`中。
最后,通过计算并集面积,即`area1[:, None] + area2 - inter`,得到了并集的面积,存储在`union`中。
最后一步,通过将交集面积除以并集面积,即`inter / union`,得到了最终的IoU值,存储在`iou`中。
函数返回了两个值,分别是IoU值和并集面积。
相关推荐
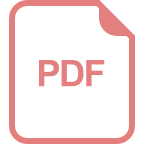
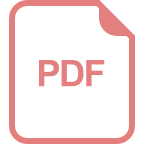
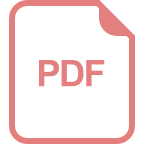













