树莓派串口怎么发送和接受多个unsigned char 程序
时间: 2023-05-26 20:07:32 浏览: 99
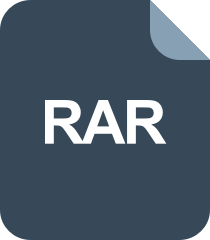
一个可以发多组的串口程序
发送和接收多个unsigned char数据,可以通过使用UART进行串口通信实现。 下面是一个简单的示例程序,演示如何使用树莓派的UART发送和接收多个unsigned char数据。
发送程序:
```
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
printf("Error opening serial port\n");
return -1;
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
//定义数据
unsigned char data[] = {0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD};
//发送数据
int len = write(fd, data, sizeof(data));
if (len != sizeof(data))
{
printf("Error writing to serial port\n");
return -1;
}
close(fd);
return 0;
}
```
接收程序:
```
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
printf("Error opening serial port\n");
return -1;
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
//定义接收缓存区
unsigned char buffer[1024];
//从串口读取数据
int len = read(fd, buffer, sizeof(buffer));
if (len == -1)
{
printf("Error reading from serial port\n");
return -1;
}
else if (len == 0)
{
printf("No data on serial port\n");
return -1;
}
//打印接收到的数据
for (int i = 0; i < len; i++)
{
printf("%02X ", buffer[i]);
}
printf("\n");
close(fd);
return 0;
}
```
上述程序中,假设使用的是树莓派3B+自带的UART串口,即/dev/ttyS0。为了进行串口通信,需要打开该串口,并设置波特率、数据位、停止位、校验位等参数。发送端使用write函数发送数据,接收端使用read函数接收数据。接收到的数据存储在接收缓存区中,通过循环遍历将其打印出来。在实际应用中,多个unsigned char数据的发送和接收可以通过定义数据结构或协议实现。
阅读全文
相关推荐


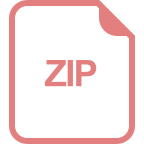
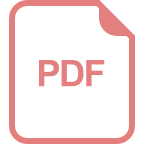
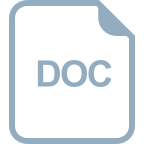









