树莓派 串口 发送多个 unsigned char
时间: 2023-05-26 19:07:35 浏览: 57
要通过串口发送多个 unsigned char,可以按照以下步骤进行:
1. 打开串口设备文件
在树莓派中,通常是通过/dev/ttyXX来访问串口设备的,其中XX表示串口编号。打开串口设备文件可以使用open函数,例如:
```
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
```
其中,O_RDWR表示以读写方式打开串口设备文件,O_NOCTTY表示不将串口设备设置为控制终端。
2. 配置串口参数
在发送数据之前,需要配置一些串口参数,例如波特率、数据位、停止位、校验位等。可以使用termios库中的函数进行配置,例如:
```
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~PARENB;
tcsetattr(fd, TCSANOW, &options);
```
其中,设置波特率为115200,数据位为8位,停止位为1位,无校验位。这里使用的是c_cflag选项,其他选项的含义可以参考文档。
3. 发送数据
在配置完串口参数后,就可以通过write函数向串口发送数据:
```
unsigned char data[] = {0x11, 0x22, 0x33};
write(fd, data, sizeof(data));
```
其中,data数组是待发送的数据,sizeof(data)表示数据的长度。
4. 关闭串口
在使用完串口之后,需要将其关闭:
```
close(fd);
```
完整代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main(void)
{
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("open");
exit(1);
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~PARENB;
tcsetattr(fd, TCSANOW, &options);
unsigned char data[] = {0x11, 0x22, 0x33};
write(fd, data, sizeof(data));
close(fd);
return 0;
}
```
相关推荐
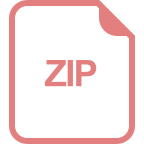
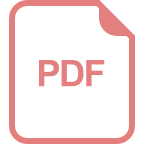
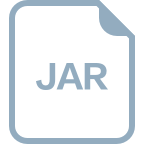














