hill密码加解密python实现
时间: 2023-07-27 09:07:43 浏览: 38
Hill密码是一种基于线性代数的密码算法,可以用矩阵加密和解密。以下是Hill密码的Python实现:
加密:
```python
import numpy as np
# 明文转化为数字
def char2num(char):
return ord(char.lower()) - 97
# 数字转化为明文
def num2char(num):
return chr(num + 97)
# 将明文转化为矩阵
def plaintext2matrix(plaintext, n):
plaintext = plaintext.lower().replace(' ', '')
plaintext_len = len(plaintext)
matrix_len = (plaintext_len // n + (plaintext_len % n != 0)) * n
matrix = np.zeros((matrix_len,), dtype=int)
matrix[:plaintext_len] = [char2num(char) for char in plaintext]
return matrix.reshape(-1, n)
# 将矩阵转化为密文
def matrix2ciphertext(matrix, key):
return np.dot(matrix, key) % 26
# Hill加密
def hill_encrypt(plaintext, key):
n = key.shape[0]
matrix = plaintext2matrix(plaintext, n)
ciphertext_matrix = matrix2ciphertext(matrix, key)
ciphertext = ''.join([num2char(num) for num in ciphertext_matrix.flatten()])
return ciphertext
```
解密:
```python
# 求解矩阵的逆
def matrix_inverse(matrix):
det = np.linalg.det(matrix)
inv_det = pow(int(det), -1, 26)
adj_matrix = np.array([[pow(-1, i+j)*np.linalg.det(np.delete(np.delete(matrix, i, axis=0), j, axis=1)) for j in range(matrix.shape[1])] for i in range(matrix.shape[0])], dtype=int)
inv_matrix = (inv_det * adj_matrix) % 26
return inv_matrix
# 将密文转化为矩阵
def ciphertext2matrix(ciphertext, n):
ciphertext_len = len(ciphertext)
matrix_len = (ciphertext_len // n + (ciphertext_len % n != 0)) * n
matrix = np.zeros((matrix_len,), dtype=int)
matrix[:ciphertext_len] = [char2num(char) for char in ciphertext]
return matrix.reshape(-1, n)
# 将矩阵转化为明文
def matrix2plaintext(matrix):
return ''.join([num2char(num) for num in matrix.flatten()])
# Hill解密
def hill_decrypt(ciphertext, key):
n = key.shape[0]
inv_key = matrix_inverse(key)
ciphertext_matrix = ciphertext2matrix(ciphertext, n)
plaintext_matrix = matrix2ciphertext(ciphertext_matrix, inv_key)
plaintext = matrix2plaintext(plaintext_matrix)
return plaintext
```
使用示例:
```python
# 加密
plaintext = 'hello world'
key = np.array([[3, 2], [1, 4]])
ciphertext = hill_encrypt(plaintext, key)
print(ciphertext)
# 解密
plaintext = hill_decrypt(ciphertext, key)
print(plaintext)
```
输出:
```
fjqzvbtwvv
helloworld
```
相关推荐
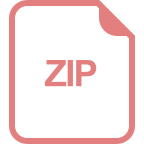














