winform调用摄像头扫描识别条形码在文本框中,完整组件及代码
时间: 2024-02-23 11:00:33 浏览: 101
这里提供一个简单的WinForm组件和代码,可以调用摄像头扫描条形码并将其识别后显示在文本框中。使用了ZXing库进行条形码识别。
组件包含一个Button和一个TextBox控件,Button用于启动摄像头扫描,TextBox用于显示识别出的条形码内容。
首先需要安装ZXing库,可以通过NuGet包管理器安装。
代码如下:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using ZXing;
namespace BarcodeScanner
{
public partial class BarcodeScannerControl : UserControl
{
private readonly Webcam webcam;
private readonly Timer timer;
private readonly BarcodeReader barcodeReader;
public BarcodeScannerControl()
{
InitializeComponent();
webcam = new Webcam();
timer = new Timer { Interval = 100 };
barcodeReader = new BarcodeReader();
}
private void buttonScan_Click(object sender, EventArgs e)
{
if (webcam.IsRunning)
{
webcam.Stop();
timer.Stop();
buttonScan.Text = "Scan";
}
else
{
webcam.Start();
timer.Start();
buttonScan.Text = "Stop";
}
}
private void timer_Tick(object sender, EventArgs e)
{
if (!webcam.IsRunning)
{
return;
}
using (var bitmap = webcam.GetBitmap())
{
if (bitmap == null)
{
return;
}
var result = barcodeReader.Decode(bitmap);
if (result == null)
{
return;
}
textBoxBarcode.Text = result.Text;
webcam.Stop();
timer.Stop();
buttonScan.Text = "Scan";
}
}
}
public class Webcam
{
private readonly VideoCaptureDevice videoCaptureDevice;
public bool IsRunning { get; private set; }
public Webcam()
{
var videoCaptureDeviceMoniker = (new FilterInfoCollection(FilterCategory.VideoInputDevice))[0].MonikerString;
videoCaptureDevice = new VideoCaptureDevice(videoCaptureDeviceMoniker);
}
public void Start()
{
videoCaptureDevice.NewFrame += VideoCaptureDevice_NewFrame;
videoCaptureDevice.Start();
IsRunning = true;
}
public void Stop()
{
videoCaptureDevice.NewFrame -= VideoCaptureDevice_NewFrame;
videoCaptureDevice.SignalToStop();
videoCaptureDevice.WaitForStop();
IsRunning = false;
}
public Bitmap GetBitmap()
{
return videoCaptureDevice.IsRunning ? (Bitmap)videoCaptureDevice.GetCurrentVideoFrame().Clone() : null;
}
private void VideoCaptureDevice_NewFrame(object sender, AForge.Video.NewFrameEventArgs eventArgs)
{
// Do nothing, just need to fire the event to obtain new frames
}
}
}
```
使用方法:
1. 新建一个WinForm应用程序,在设计视图中添加一个UserControl控件。
2. 在UserControl中添加一个Button和一个TextBox控件。
3. 将以上代码复制到UserControl的.cs文件中,并将命名空间改成你的应用程序命名空间。
4. 在UserControl中添加以下代码:
```csharp
private readonly BarcodeScannerControl barcodeScannerControl;
public MainForm()
{
InitializeComponent();
barcodeScannerControl = new BarcodeScannerControl();
Controls.Add(barcodeScannerControl);
barcodeScannerControl.Dock = DockStyle.Fill;
}
```
5. 编译并运行程序,点击Button开始扫描条形码。扫描到条形码后,识别结果会显示在TextBox中。
阅读全文
相关推荐
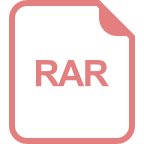





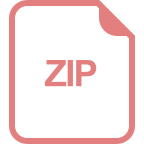
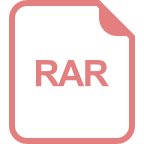
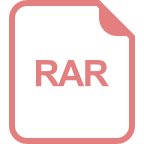
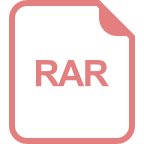
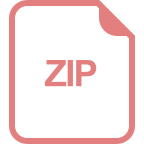
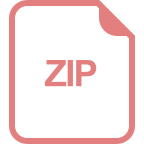
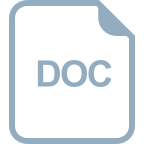


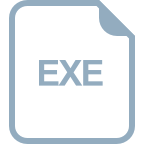
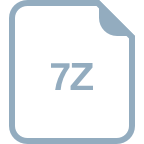