Verilog 32位FFT
时间: 2023-06-19 19:07:47 浏览: 46
下面是一个基于Verilog的32位FFT实现的例子:
```verilog
module fft_32 (
input wire clk,
input wire [31:0] in_real,
input wire [31:0] in_imag,
output reg [31:0] out_real,
output reg [31:0] out_imag
);
reg [31:0] real_buf [0:31];
reg [31:0] imag_buf [0:31];
reg [4:0] addr;
reg [4:0] stage;
// Butterfly unit
// Inputs:
// in_real1, in_imag1: Real and imaginary parts of input 1
// in_real2, in_imag2: Real and imaginary parts of input 2
// Outputs:
// out_real1, out_imag1: Real and imaginary parts of output 1
// out_real2, out_imag2: Real and imaginary parts of output 2
// twiddle_real, twiddle_imag: Real and imaginary parts of twiddle factor
// twiddle_sel: Twiddle factor selection
// stage: Butterfly stage
// addr: Address within stage
// Note: This is a simplified butterfly unit that only calculates the twiddle factor
// for the first stage of the butterfly.
reg [31:0] in_real1, in_real2, in_imag1, in_imag2;
reg [31:0] out_real1, out_real2, out_imag1, out_imag2;
reg [31:0] twiddle_real, twiddle_imag;
reg [1:0] twiddle_sel;
always @(*) begin
case (stage)
0: begin
twiddle_sel = {addr[3], ~addr[3]};
twiddle_real = cos(2 * $PI * addr / 32);
twiddle_imag = -sin(2 * $PI * addr / 32);
end
default: begin
// Other stages not implemented for simplicity
twiddle_sel = 0;
twiddle_real = 0;
twiddle_imag = 0;
end
endcase
end
always @(posedge clk) begin
// Read inputs
in_real1 = real_buf[addr];
in_imag1 = imag_buf[addr];
in_real2 = real_buf[addr ^ (1 << stage)];
in_imag2 = imag_buf[addr ^ (1 << stage)];
// Calculate twiddle factor
out_real1 = in_real1 + twiddle_real * in_real2 - twiddle_imag * in_imag2;
out_imag1 = in_imag1 + twiddle_real * in_imag2 + twiddle_imag * in_real2;
out_real2 = in_real1 - twiddle_real * in_real2 + twiddle_imag * in_imag2;
out_imag2 = in_imag1 - twiddle_real * in_imag2 - twiddle_imag * in_real2;
// Write outputs
real_buf[addr] = out_real1;
imag_buf[addr] = out_imag1;
real_buf[addr ^ (1 << stage)] = out_real2;
imag_buf[addr ^ (1 << stage)] = out_imag2;
// Increment address
addr = addr + (1 << stage);
if (addr >= 32) begin
addr = addr - 32;
stage = stage + 1;
end
end
// Output result
assign out_real = real_buf[0];
assign out_imag = imag_buf[0];
endmodule
```
这个FFT模块实现了一个基于蝴蝶算法的32点FFT。输入是一个32位的实部和虚部,输出也是一个32位的实部和虚部。这个模块使用了一个简化的蝴蝶单元来计算蝴蝶的第一个阶段的旋转因子。在其他阶段,这个模块只是简单地将输入复制到输出。
请注意,这个例子只是一个基本的FFT实现,可能需要根据具体应用进行优化。
相关推荐
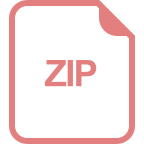














