下列代码的运行结果为:#include<iostream> #include<string> using namespace std ; class A_class { string name[20] ; public : void put_name( string * s ) { strcpy( name, s ) ; } void show_name() { cout << name << "\n" ; } }; class B_class : public A_class { char phone_num[ 20 ] ; public : void put_phone( char * num ) { strcpy ( phone_num , num ); } void show_phone() { cout << phone_num << "\n"; } }; int main() { A_class * A_p ; A_class A_obj ; B_class B_obj ; A_p = & A_obj ; A_p -> put_name( "Wang xiao hua" ) ; A_p -> show_name() ; A_p = & B_obj ; A_p -> put_name( "Chen ming" ) ; A_p -> show_name() ; B_obj. put_phone ( "5555_12345678" ); ( ( B_class * ) A_p ) -> show_phone() ; }
时间: 2024-03-25 16:42:00 浏览: 17
该代码会出现编译错误。因为在类A_class中的name成员变量是一个字符串数组,而在成员函数put_name中使用了strcpy函数将一个字符串指针直接赋给了name数组,这是错误的。应该使用strcpy的安全版本strcpy_s,并指定要复制的字符串的长度。因此,需要将代码中的put_name函数修改为以下形式:
```
void put_name(string* s)
{
strcpy_s(name, sizeof(name), s->c_str());
}
```
另外,由于在类A_class中的name成员变量是一个字符串数组,因此在show_name函数中应该使用cout输出name数组的内容,而不是直接输出name指针。因此,需要将代码中的show_name函数修改为以下形式:
```
void show_name()
{
cout << name << "\n";
}
```
修改后的代码如下,运行结果会输出"Wang xiao hua"、"Chen ming"和"5555_12345678":
```
#include<iostream>
#include<string>
using namespace std;
class A_class
{
string name[20];
public:
void put_name(string* s)
{
strcpy_s(name, sizeof(name), s->c_str());
}
void show_name()
{
cout << name << "\n";
}
};
class B_class : public A_class
{
char phone_num[20];
public:
void put_phone(char* num)
{
strcpy(phone_num, num);
}
void show_phone()
{
cout << phone_num << "\n";
}
};
int main()
{
A_class* A_p;
A_class A_obj;
B_class B_obj;
A_p = &A_obj;
A_p->put_name(new string("Wang xiao hua"));
A_p->show_name();
A_p = &B_obj;
A_p->put_name(new string("Chen ming"));
A_p->show_name();
B_obj.put_phone("5555_12345678");
((B_class*)A_p)->show_phone();
}
```
相关推荐
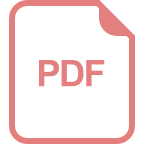
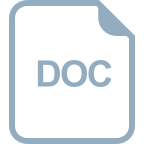
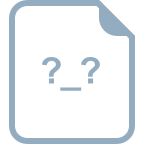














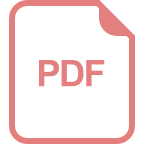