3.c #include <stdio.h> #include <stdlib.h> #include <pthread.h> int sum1 = 0, sum2 = 0; void *p1(){ int i, tmp = 0; for (i = 1; i <= 100; i++) tmp += i; sum1 += tmp; } void p2(){ int i, tmp = 0; for (i = 101; i <= 200; i++) tmp += i; sum2 += tmp; } void p3(){ printf("sum: %d\n", sum1 + sum2); } int main(){ int res; pthread_t t; void *thread_result; res = pthread_create(&t, NULL, p1, NULL); if (res != 0){ perror("failed to create thread"); exit(1); } p2(); res = pthread_join(t, &thread_result); if (res != 0){ perror("failed to join thread"); exit(2); } p3(); return 0; } 什么意思
时间: 2024-04-23 20:25:42 浏览: 109
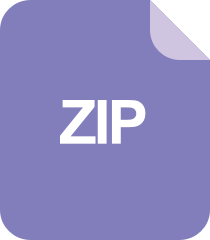
SeqList.h #include <stdio.h> //包含标准输入输出流的头文件 #include <assert.h
这是一个使用 pthread 线程库的 C 语言程序,它创建了一个线程(p1)和两个函数(p2 和 p3)来计算并输出 1~200 的所有整数之和。在主函数中,它首先创建线程 p1,然后调用函数 p2 来计算 101~200 的整数和,最后等待线程 p1 执行完成后,调用函数 p3 来输出总和。需要注意的是,sum1 和 sum2 是在主函数和线程函数之间共享的全局变量,因此需要使用互斥锁等机制来保证线程安全。
阅读全文
相关推荐
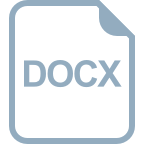















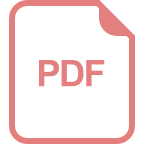