% Example usage A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; b = [1; 2; 3]; [L, U] = lu_decomposition(A); y = forward_substitution(L, b); x = backward_substitution(U, y);
时间: 2024-04-28 22:20:14 浏览: 35
这是一个使用 LU 分解解线性方程组的例子。LU 分解可以将一个矩阵分解为一个下三角矩阵 L 和一个上三角矩阵 U 的乘积,因此可以用来求解线性方程组 Ax = b,其中 A 是一个方阵,b 是一个列向量。
在这个例子中,矩阵 A 是一个 $3 \times 3$ 的矩阵,列向量 b 是一个 $3 \times 1$ 的向量。首先,使用 lu_decomposition 函数对 A 进行 LU 分解,得到 L 和 U 两个矩阵。然后,使用 forward_substitution 函数对 Ly=b 进行前向代入,得到向量 y。最后,使用 backward_substitution 函数对 Ux=y 进行后向代入,得到向量 x,它就是线性方程组的解。
注意,这里的 LU 分解和前向/后向代入都需要使用特定的算法来实现,具体实现方法可以参考相关的数值分析书籍或者在线资源。
相关问题
Given two singly linked lists L 1 =a 1 →a 2 →⋯→a n−1 →a n and L 2 =b 1 →b 2 →⋯→b m−1 →b m . If n≥2m, you are supposed to reverse and merge the shorter one into the longer one to obtain a list like a 1 →a 2 →b m →a 3 →a 4 →b m−1 ⋯. For example, given one list being 6→7 and the other one 1→2→3→4→5, you must output 1→2→7→3→4→6→5.
To solve this problem, we can first find out which list is shorter and which is longer. Then we can reverse the shorter list and merge it into the longer list. Here's the step-by-step process:
1. Check which list is shorter and which is longer. Let's assume L1 is shorter and L2 is longer.
2. Reverse the shorter list L1. This can be done by iterating through the list and changing the pointers of each node to point to the previous node instead of the next node.
3. Merge the two lists by iterating through both lists at the same time. For each element, we compare the current node of L1 with the current node of L2. If the current node of L1 is smaller, we add it to the merged list and move on to the next node in L1. If the current node of L2 is smaller, we add it to the merged list and move on to the next node in L2.
4. If we reach the end of either list, we simply add the remaining nodes of the other list to the merged list.
5. Finally, we reverse the shorter list L1 again to restore its original order.
Here's the Python code:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_list(head):
prev = None
curr = head
while curr:
next_node = curr.next
curr.next = prev
prev = curr
curr = next_node
return prev
def merge_lists(l1, l2):
dummy = ListNode()
curr = dummy
while l1 and l2:
if l1.val < l2.val:
curr.next = l1
l1 = l1.next
else:
curr.next = l2
l2 = l2.next
curr = curr.next
if l1:
curr.next = l1
if l2:
curr.next = l2
return dummy.next
def merge_reverse_lists(l1, l2):
if not l1 or not l2:
return l1 or l2
if length(l1) < length(l2):
l1, l2 = l2, l1
l1_rev = reverse_list(l1)
merged = merge_lists(l1_rev, l2)
l1_rev_rev = reverse_list(l1_rev)
return merge_lists(l1_rev_rev, merged)
def length(head):
count = 0
while head:
count += 1
head = head.next
return count
```
You can use this code to test the solution:
```python
# Example usage
l1 = ListNode(6, ListNode(7))
l2 = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))
merged = merge_reverse_lists(l1, l2)
while merged:
print(merged.val, end=' ')
merged = merged.next
# Output: 1 2 7 3 4 6 5
```
修改代码格式 import random def gcd(a, b): if b == 0: return a else: return gcd(b, a % b) def mod_inverse(a, m): for x in range(1, m): if (a*x) % m == 1: return x return None def blind_sign(m, n, e, d): # Generate random value r r = random.randint(1, n-1) while gcd(r, n) != 1: r = random.randint(1, n-1) # Blind message m_blind = (m * pow(r, e)) % n # Sign blinded message s_blind = pow(m_blind, d) % n # Unblind signed message s = (s_blind * mod_inverse(r, n)) % n return s # Example usage n = 3233 # modulus e = 17 # public exponent d = 2753 # private exponent m = 1234 # message to be signed s = blind_sign(m, n, e, d) print("Message:", m) print("Signature:", s)
修改后的代码格式如下:
import random
def gcd(a, b):
if b == 0:
return a
else:
return gcd(b, a % b)
def mod_inverse(a, m):
for x in range(1, m):
if (a*x) % m == 1:
return x
return None
def blind_sign(m, n, e, d):
# Generate random value r
r = random.randint(1, n-1)
while gcd(r, n) != 1:
r = random.randint(1, n-1)
# Blind message
m_blind = (m * pow(r, e)) % n
# Sign blinded message
s_blind = pow(m_blind, d) % n
# Unblind signed message
s = (s_blind * mod_inverse(r, n)) % n
return s
# Example usage
n = 3233 # modulus
e = 17 # public exponent
d = 2753 # private exponent
m = 1234 # message to be signed
s = blind_sign(m, n, e, d)
print("Message:", m)
print("Signature:", s)
阅读全文
相关推荐
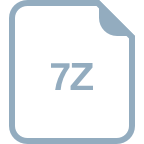
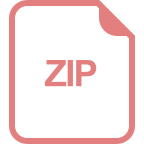
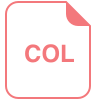
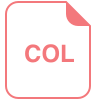












