A*算法中cost函数是什么
时间: 2024-04-02 07:37:22 浏览: 13
在 A* 算法中,cost 函数指的是从一个节点到另一个节点的代价,也称路径代价。该代价通常包括两个部分:一个是从起点到当前节点的实际代价(即 g(n) 值),另一个是从当前节点到目标节点的估计代价(即 h(n) 值)。因此,cost(n1, n2) = g(n1) + h(n2),其中 n1 和 n2 分别表示两个节点。
其中,g(n) 表示从起点到当前节点 n 的实际代价,可以通过计算从起点到当前节点的路径长度得到。而 h(n) 表示从当前节点 n 到目标节点的估计代价,通常使用启发式函数来计算。常见的启发式函数有曼哈顿距离、欧几里得距离、切比雪夫距离等。
因此,A* 算法中的 cost 函数可以理解为一个综合考虑了实际代价和估计代价的路径代价函数。
相关问题
如何修改matlab A*算法代价函数
在Matlab中,可以通过修改代价函数来改变A*算法的行为。代价函数是一个计算从起点到当前节点的代价的函数。具体来说,它将当前节点与起点之间的距离加上当前节点的启发式估计值,以计算当前节点的总代价。启发式估计值是从当前节点到目标节点的估计距离,通常使用曼哈顿距离或欧几里得距离来计算。
以下是一个简单的A*算法的代码示例,其中代价函数被定义为距离加上启发式估计值:
```matlab
function [path, cost] = astar(startNode, goalNode, adjMatrix, heuristic)
% Initialize the algorithm
visited = false(size(adjMatrix, 1), 1);
gScore = Inf(size(adjMatrix, 1), 1);
fScore = Inf(size(adjMatrix, 1), 1);
gScore(startNode) = 0;
fScore(startNode) = heuristic(startNode, goalNode);
% Search for the goal node
while ~all(visited)
% Find the node with the lowest fScore
[~, current] = min(fScore(~visited));
visited(current) = true;
% Check if we've reached the goal node
if current == goalNode
path = backtrackPath(goalNode);
cost = gScore(goalNode);
return
end
% Update the gScore and fScore of neighbors
neighbors = find(adjMatrix(current, :));
for i = 1:length(neighbors)
neighbor = neighbors(i);
if visited(neighbor)
continue
end
tentativeGScore = gScore(current) + adjMatrix(current, neighbor);
if tentativeGScore < gScore(neighbor)
gScore(neighbor) = tentativeGScore;
fScore(neighbor) = gScore(neighbor) + heuristic(neighbor, goalNode);
end
end
end
% If we get here, there's no path to the goal node
path = [];
cost = Inf;
end
function path = backtrackPath(node)
% Backtrack from the goal node to the start node
path = node;
while ~isempty(node.parent)
node = node.parent;
path = [node path];
end
end
```
如果您想修改代价函数,只需更改启发式估计值的计算方式即可。例如,如果您想使用曼哈顿距离作为启发式估计值,可以将启发式函数定义为:
```matlab
function h = manhattanDistance(node, goalNode)
% Compute the Manhattan distance between node and goalNode
dx = abs(node.x - goalNode.x);
dy = abs(node.y - goalNode.y);
h = dx + dy;
end
```
然后,在调用A*算法时,将启发式函数作为参数传递:
```matlab
path = astar(startNode, goalNode, adjMatrix, @manhattanDistance);
```
您可以根据需要修改代价函数,以适应不同的问题和场景。
matlab中三维A*算法
A*算法是一种在图形搜索和路径规划中广泛应用的启发式搜索算法。它可以用于三维路径规划,但是需要对算法进行适当的修改。
在三维A*算法中,需要将搜索空间从平面扩展到立体空间。这可以通过将每个节点表示为三元组(x,y,z),其中x、y、z分别表示节点在三个轴上的坐标来实现。
在实现A*算法时,需要确定合适的启发式函数来估计从当前节点到目标节点的距离。在三维空间中,可以使用欧几里得距离或曼哈顿距离等距离函数作为启发式函数。
另外,还需要考虑如何表示障碍物。一种常见的方法是将三维空间划分为立方体网格,并将每个网格标记为障碍或非障碍。在搜索过程中,需要避开障碍物。
以下是一个简单的三维A*算法的示例代码:
```
function [path, cost] = astar3d(map, start, goal)
% Three-dimensional A* algorithm
% map: 3D occupancy grid (1: free, 0: occupied)
% start: starting position (3D coordinate)
% goal: goal position (3D coordinate)
% Define heuristic function (Euclidean distance)
heuristic = @(pos) norm(pos - goal);
% Initialize open and closed lists
open = PriorityQueue();
closed = containers.Map();
% Add starting node to open list
g_score = 0;
f_score = g_score + heuristic(start);
open.insert(start, f_score);
% Loop until goal is found or open list is empty
while ~open.isempty()
% Get node with lowest f-score from open list
[current_pos, f_score] = open.pop();
% Check if current node is goal
if isequal(current_pos, goal)
% Reconstruct path and return
path = reconstruct_path(closed, start, goal);
cost = g_score;
return;
end
% Add current node to closed list
closed(num2str(current_pos)) = g_score;
% Expand neighbors
neighbors = get_neighbors(map, current_pos);
for i = 1:size(neighbors, 1)
neighbor_pos = neighbors(i, :);
% Calculate tentative g-score for neighbor
tentative_g_score = g_score + norm(current_pos - neighbor_pos);
% Check if neighbor is already in closed list
if isKey(closed, num2str(neighbor_pos))
% Skip neighbor if it has already been evaluated
continue;
end
% Check if neighbor is in open list
if open.ismember(neighbor_pos)
% Check if tentative g-score is better than previous g-score
if tentative_g_score < closed(num2str(neighbor_pos))
% Update neighbor's g-score and f-score
closed(num2str(neighbor_pos)) = tentative_g_score;
f_score = tentative_g_score + heuristic(neighbor_pos);
open.update(neighbor_pos, f_score);
end
else
% Add neighbor to open list
closed(num2str(neighbor_pos)) = tentative_g_score;
f_score = tentative_g_score + heuristic(neighbor_pos);
open.insert(neighbor_pos, f_score);
end
end
% Update g-score
g_score = closed(num2str(current_pos));
end
% No path found
path = [];
cost = inf;
end
function neighbors = get_neighbors(map, pos)
% Get neighboring nodes that are free and within map bounds
[x, y, z] = ind2sub(size(map), find(map));
neighbors = [x, y, z];
neighbors = neighbors(~ismember(neighbors, pos, 'rows'), :);
distances = pdist2(pos, neighbors);
neighbors(distances > sqrt(3)) = NaN; % limit to 1-neighborhood
neighbors(any(isnan(neighbors), 2), :) = [];
neighbors = neighbors(map(sub2ind(size(map), neighbors(:,1), neighbors(:,2), neighbors(:,3))) == 1, :);
end
function path = reconstruct_path(closed, start, goal)
% Reconstruct path from closed list
path = [goal];
while ~isequal(path(1,:), start)
pos = path(1,:);
for dx = -1:1
for dy = -1:1
for dz = -1:1
neighbor_pos = pos + [dx, dy, dz];
if isKey(closed, num2str(neighbor_pos)) && closed(num2str(neighbor_pos)) < closed(num2str(pos))
pos = neighbor_pos;
end
end
end
end
path = [pos; path];
end
end
classdef PriorityQueue < handle
% Priority queue implemented as binary heap
properties (Access = private)
heap;
count;
end
methods
function obj = PriorityQueue()
obj.heap = {};
obj.count = 0;
end
function insert(obj, item, priority)
% Add item with given priority to queue
obj.count = obj.count + 1;
obj.heap{obj.count} = {item, priority};
obj.sift_up(obj.count);
end
function [item, priority] = pop(obj)
% Remove and return item with lowest priority from queue
item = obj.heap{1}{1};
priority = obj.heap{1}{2};
obj.heap{1} = obj.heap{obj.count};
obj.count = obj.count - 1;
obj.sift_down(1);
end
function update(obj, item, priority)
% Update priority of given item in queue
for i = 1:obj.count
if isequal(obj.heap{i}{1}, item)
obj.heap{i}{2} = priority;
obj.sift_up(i);
break;
end
end
end
function tf = isempty(obj)
% Check if queue is empty
tf = obj.count == 0;
end
function tf = ismember(obj, item)
% Check if item is in queue
tf = false;
for i = 1:obj.count
if isequal(obj.heap{i}{1}, item)
tf = true;
break;
end
end
end
end
methods (Access = private)
function sift_up(obj, index)
% Move item up in heap until it satisfies heap property
while index > 1
parent_index = floor(index / 2);
if obj.heap{index}{2} < obj.heap{parent_index}{2}
temp = obj.heap{index};
obj.heap{index} = obj.heap{parent_index};
obj.heap{parent_index} = temp;
index = parent_index;
else
break;
end
end
end
function sift_down(obj, index)
% Move item down in heap until it satisfies heap property
while index * 2 <= obj.count
child_index = index * 2;
if child_index + 1 <= obj.count && obj.heap{child_index + 1}{2} < obj.heap{child_index}{2}
child_index = child_index + 1;
end
if obj.heap{child_index}{2} < obj.heap{index}{2}
temp = obj.heap{index};
obj.heap{index} = obj.heap{child_index};
obj.heap{child_index} = temp;
index = child_index;
else
break;
end
end
end
end
end
```
该实现使用了一个基于二叉堆的优先队列来管理开放列表,并使用容器映射来管理关闭列表。搜索空间被划分为立方体网格,并使用三元组(x,y,z)表示每个节点的位置。启发式函数使用欧几里得距离,障碍物被标记为0,空闲区域被标记为1。在搜索过程中,只扩展空闲节点,并且避开障碍物。
请注意,该实现并不是最优的实现,因为它没有使用任何优化技巧,如跳跃点或平滑路径。但是,它可以作为三维A*算法的一个简单示例来帮助您开始。
相关推荐
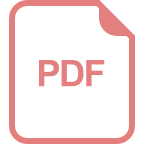
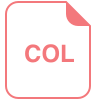
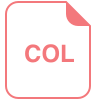
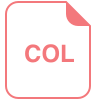









