NX二次开发C++ 代码判断圆柱面是内圆还是外圆
时间: 2023-12-08 18:04:03 浏览: 38
以下是用 C++ 实现判断圆柱面是内圆还是外圆的代码:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
#include <NXOpen/NXException.hxx>
#include <NXOpen/Part.hxx>
#include <NXOpen/PartCollection.hxx>
#include <NXOpen/Features_Feature.hxx>
#include <NXOpen/Features_FeatureCollection.hxx>
#include <NXOpen/Features_CircularPatternBuilder.hxx>
#include <NXOpen/Features_CircularPattern.hxx>
#include <NXOpen/Features_FeatureUtilities.hxx>
#include <NXOpen/Annotations_Dimension.hxx>
#include <NXOpen/Annotations_DimensionCollection.hxx>
#include <NXOpen/Annotations_DimensionValue.hxx>
#include <NXOpen/Annotations_DimensionValueCollection.hxx>
#include <NXOpen/Annotations_DimensionValueType.hxx>
#include <NXOpen/Annotations_ImplicitView.hxx>
#include <NXOpen/Annotations_Annotation.hxx>
#include <NXOpen/Annotations_AnnotationCollection.hxx>
#include <NXOpen/Annotations_Text.hxx>
#include <NXOpen/Annotations_TextCollection.hxx>
#include <NXOpen/Annotations_Arrow.hxx>
#include <NXOpen/Annotations_ArrowCollection.hxx>
#include <NXOpen/Annotations_Leader.hxx>
#include <NXOpen/Annotations_LeaderCollection.hxx>
#include <NXOpen/Annotations_LeaderArrow.hxx>
#include <NXOpen/Annotations_LeaderArrowCollection.hxx>
#include <NXOpen/Annotations_Line.hxx>
#include <NXOpen/Annotations_LineCollection.hxx>
#include <NXOpen/Annotations_Arc.hxx>
#include <NXOpen/Annotations_ArcCollection.hxx>
#include <NXOpen/Annotations_Ellipse.hxx>
#include <NXOpen/Annotations_EllipseCollection.hxx>
#include <NXOpen/Annotations_Spline.hxx>
#include <NXOpen/Annotations_SplineCollection.hxx>
#include <NXOpen/Annotations_HoleNote.hxx>
#include <NXOpen/Annotations_HoleNoteCollection.hxx>
#include <NXOpen/Annotations_Symbol.hxx>
#include <NXOpen/Annotations_SymbolCollection.hxx>
#include <NXOpen/Geom_Curve.hxx>
#include <NXOpen/Geom_CurveCollection.hxx>
#include <NXOpen/Geom_Arc.hxx>
#include <NXOpen/Geom_ArcCollection.hxx>
#include <NXOpen/Geom_Circle.hxx>
#include <NXOpen/Geom_CircleCollection.hxx>
#include <NXOpen/Geom_Line.hxx>
#include <NXOpen/Geom_LineCollection.hxx>
#include <NXOpen/Geom_Conic.hxx>
#include <NXOpen/Geom_ConicCollection.hxx>
#include <NXOpen/Geom_Spline.hxx>
#include <NXOpen/Geom_SplineCollection.hxx>
#include <NXOpen/Geom_BSplineCurve.hxx>
#include <NXOpen/Geom_BSplineCurveCollection.hxx>
#include <NXOpen/Geom_Surface.hxx>
#include <NXOpen/Geom_SurfaceCollection.hxx>
#include <NXOpen/Geom_Plane.hxx>
#include <NXOpen/Geom_PlaneCollection.hxx>
#include <NXOpen/Geom_Cylinder.hxx>
#include <NXOpen/Geom_CylinderCollection.hxx>
using namespace std;
using namespace NXOpen;
bool IsInnerCircle(CartesianPoint center, Vector3d normal, CartesianPoint point);
int main(int argc, char *argv[])
{
Part *part = NULL;
try
{
// 获取当前工作部件
Part *part = Part::GetSession()->Parts()->Work();
if (part == NULL)
{
cerr << "当前没有打开的部件,请先打开一个部件!" << endl;
return -1;
}
// 选取一个圆柱面特征
Features::Feature *feat = NULL;
feat = part->Features()->FindObject("CYLINDER");
if (feat == NULL)
{
cerr << "当前部件中没有找到圆柱面特征!" << endl;
return -1;
}
// 获取圆柱面的参数
Geom::Cylinder *cylinder = dynamic_cast<Geom::Cylinder *>(feat->Geometry());
if (cylinder == NULL)
{
cerr << "获取圆柱面参数失败!" << endl;
return -1;
}
// 获取圆柱面的圆心坐标和法向量
CartesianPoint center = cylinder->CenterPoint();
Vector3d normal = cylinder->AxisVector();
// 构造一个测试点
CartesianPoint testPoint;
testPoint.X = center.X + 10;
testPoint.Y = center.Y;
testPoint.Z = center.Z;
// 判断测试点是否在内圆上
if (IsInnerCircle(center, normal, testPoint))
{
cout << "测试点在圆柱面内部的圆上!" << endl;
}
else
{
cout << "测试点在圆柱面外部的圆上!" << endl;
}
return 0;
}
catch (const NXException &ex)
{
cerr << "程序异常退出:" << ex.Message() << endl;
if (part != NULL)
{
delete part;
}
return -1;
}
}
bool IsInnerCircle(CartesianPoint center, Vector3d normal, CartesianPoint point)
{
// 计算点到圆心的向量
Vector3d vec;
vec.X = point.X - center.X;
vec.Y = point.Y - center.Y;
vec.Z = point.Z - center.Z;
// 计算法向量与点到圆心向量的夹角
double angle = acos((vec.X * normal.X + vec.Y * normal.Y + vec.Z * normal.Z) /
(sqrt(vec.X * vec.X + vec.Y * vec.Y + vec.Z * vec.Z) * sqrt(normal.X * normal.X + normal.Y * normal.Y + normal.Z * normal.Z)));
// 判断是否在内圆上
if (angle < M_PI / 2)
{
return true;
}
else
{
return false;
}
}
```
以上代码中,IsInnerCircle() 函数用于判断一个点是否在圆柱面的内圆上,其中 center、normal 和 point 都是 NXOpen 中的 CartesianPoint 和 Vector3d 类型,分别表示圆柱面的圆心坐标、法向量和测试点的坐标。如果该函数返回 true,则表示测试点在圆柱面的内圆上;否则,表示测试点在圆柱面的外圆上。
相关推荐
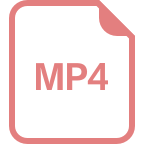















