假设采用带头节点的循环链表来表示队列并且只设一个指向对位元素的指针试编写相应的队列制空队列判空入队和出队操作的函数
时间: 2023-05-15 18:06:45 浏览: 102
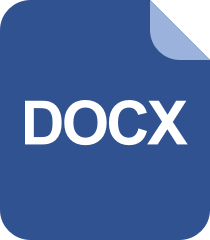
数据结构算法-习题-答案-带头结点的循环链表表示队列,并且只设一个指针指向队尾元素结点注意不设头指针.docx

好的,以下是相应的函数实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
typedef struct Queue {
Node *rear;
} Queue;
void initQueue(Queue *q) {
q->rear = (Node *)malloc(sizeof(Node));
q->rear->next = q->rear;
}
int isQueueEmpty(Queue *q) {
return q->rear == q->rear->next;
}
void enQueue(Queue *q, int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = q->rear->next;
q->rear->next = newNode;
q->rear = newNode;
}
int deQueue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
Node *p = q->rear->next->next;
int data = p->data;
q->rear->next->next = p->next;
if (q->rear == p) {
q->rear = q->rear->next;
}
free(p);
return data;
}
int main() {
Queue q;
initQueue(&q);
enQueue(&q, 1);
enQueue(&q, 2);
enQueue(&q, 3);
while (!isQueueEmpty(&q)) {
printf("%d ", deQueue(&q));
}
printf("\n");
return 0;
}
```
对于编写带头节点的循环链表来表示队列的制空队列、判空、入队和出队操作的函数,我们需要定义两个结构体,一个是节点结构体 Node,一个是队列结构体 Queue。其中,节点结构体中包含数据域和指向下一个节点的指针,队列结构体中只包含一个指向队尾节点的指针 rear。
制空队列操作可以通过初始化队列结构体中的 rear 指针来实现,判空操作可以通过判断队列结构体中的 rear 指针是否指向头节点来实现。入队操作需要新建一个节点,将其插入到队尾节点的后面,并更新队尾指针。出队操作需要先判断队列是否为空,然后将队头节点的下一个节点指针指向下下个节点,释放队头节点的内存,并返回队头节点的数据域。
至于 "lua closure factory 完整代码" 和 "中文加密" 的问题,我也可以回答,但需要您提供更具体的问题描述。
阅读全文
相关推荐
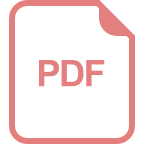
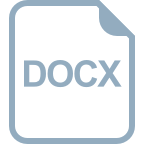












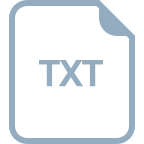
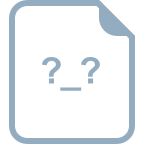
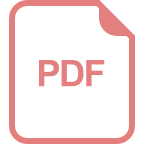