using System.Collections; using System.Collections.Generic; using UnityEditor; using UnityEngine; public class PatternSystemEditor : EditorWindow { [MenuItem("Window/AddPatternToSystem")] static void AddPatternToSystem() { var gameManager = GameObject.Find("GameManager"); if (gameManager != null) { var patternManager = gameManager.GetComponent<PatternManager>(); if (Selection.gameObjects.Length == 1) { var item = Selection.gameObjects[0].transform.Find("Item"); if (item != null) { Pattern pattern = new Pattern(); foreach (var child in item) { Transform childTransform = child as Transform; if (childTransform != null) { var prefeb = UnityEditor.PrefabUtility.GetPrefabParent(childTransform.gameObject); if (prefeb != null) { PatternItem patternItem = new PatternItem { gameobject = prefeb as GameObject, position = childTransform.localPosition }; pattern.PatternItems.Add(patternItem); } } } patternManager.Patterns.Add(pattern); } } } } }
时间: 2024-02-10 17:19:02 浏览: 124
这是一个Unity编辑器脚本,用于添加游戏中的Pattern(模式)到PatternManager(模式管理器)中。它包含一个名为AddPatternToSystem()的静态方法,该方法可以通过Unity编辑器中的菜单栏中的"Window/AddPatternToSystem"进行调用。在方法中,首先查找名为"GameManager"的游戏物体,然后获取PatternManager组件。接下来,判断当前选中的游戏物体是否为一个包含名为"Item"的子对象的物体。如果是,就创建一个新的Pattern对象,并将其子物体中的每个Prefab及其位置信息添加到PatternItems列表中。最后将Pattern添加到PatternManager的Patterns列表中。这个脚本可以方便地添加游戏中的Pattern,减少手动操作的工作量。
相关问题
unity 里写一个一键打包脚本,使导出的工程包里有libil2cpp.so文件
好的,以下是一键打包脚本示例,可供参考:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEditor;
using UnityEditor.Build.Reporting;
using System.IO;
public class BuildScript : MonoBehaviour
{
[MenuItem("Build/Build Android")]
public static void BuildAndroid()
{
// 定义导出路径和文件名
string exportPath = "Export/Android";
string exportName = "MyGame.apk";
string fullPath = Path.Combine(Application.dataPath, "../", exportPath, exportName);
// 设置 BuildPlayerOptions
BuildPlayerOptions buildPlayerOptions = new BuildPlayerOptions();
buildPlayerOptions.scenes = new[] { "Assets/Scenes/Main.unity" };
buildPlayerOptions.locationPathName = fullPath;
buildPlayerOptions.target = BuildTarget.Android;
buildPlayerOptions.options = BuildOptions.None;
// 设置 PlayerSettings
PlayerSettings.SetScriptingBackend(BuildTargetGroup.Android, ScriptingImplementation.IL2CPP);
// 执行打包
BuildReport report = BuildPipeline.BuildPlayer(buildPlayerOptions);
// 检查打包结果
if (report.summary.result != BuildResult.Succeeded)
{
Debug.LogError("Build failed!");
return;
}
// 移动 libil2cpp.so 文件到导出目录
string libil2cppPath = Path.Combine(Application.dataPath, "../", "Temp/StagingArea/libs/arm64-v8a/libil2cpp.so");
string exportLibil2cppPath = Path.Combine(Application.dataPath, "../", exportPath, "libil2cpp.so");
File.Copy(libil2cppPath, exportLibil2cppPath, true);
// 打印打包成功信息
Debug.Log("Build succeeded: " + fullPath);
}
}
```
这个脚本做了以下几件事情:
1. 定义了导出路径和文件名;
2. 设置了 BuildPlayerOptions,指定了要打包的场景、导出路径和平台等信息;
3. 设置了 PlayerSettings,将脚本后端设置为 IL2CPP;
4. 执行打包;
5. 检查打包结果,如果失败则返回;
6. 将 libil2cpp.so 文件从 Temp/StagingArea 目录移动到导出目录;
7. 打印打包成功信息。
其中第 6 步是实现你要求的功能,将 libil2cpp.so 文件移动到导出目录。注意,这个脚本中假设了目标平台是 Android,如果你需要打包到其他平台,需要相应地修改代码。
unity 获取build中的场景
在Unity中,要获取构建(Build)中的场景信息,可以在构建过程中使用脚本来记录场景信息,或者在构建后读取构建文件(如Android的APK文件)中的相关元数据。以下是一个简单的例子,展示了如何在Unity中使用脚本来获取Build中的场景信息:
1. 在Unity编辑器中,你可以创建一个脚本,并在其中使用`EditorBuildSettings`类来获取场景信息。这个类提供了编辑器中当前构建设置的所有场景列表。以下是一个简单的脚本示例:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEditor;
using System.IO;
public class BuildInfo : EditorWindow
{
[MenuItem("Tools/Show Build Info")]
public static void ShowWindow()
{
// 获取当前窗口或创建一个新窗口
GetWindow(typeof(BuildInfo));
}
void OnGUI()
{
GUILayout.Label("Build Scenes:", EditorStyles.boldLabel);
// 获取当前的Build Settings
EditorBuildSettingsScene[] scenes = EditorBuildSettings.scenes;
foreach (var scene in scenes)
{
// 这里会显示所有被勾选的场景
GUILayout.Label(Path.GetFileNameWithoutExtension(scene.path));
}
}
}
```
在上面的代码中,`EditorBuildSettings.scenes`返回一个`EditorBuildSettingsScene`数组,它包含了所有被勾选的场景的路径。这段脚本提供了一个编辑器窗口,可以在Unity编辑器中通过菜单项(Tools/Show Build Info)来打开,并显示所有构建设置中的场景。
2. 如果你想要获取的是构建后生成的文件中的场景信息,那么通常需要使用外部工具或程序来读取这些文件(如APK),解析出场景信息。对于APK文件,你可以使用反编译工具,如`apktool`,来解包APK文件,然后检查其`resources.arsc`文件中的元数据来获取场景信息。
3. 请注意,获取构建后的APK文件中的场景信息可能需要一些对Android构建过程和APK文件结构的深入了解。
阅读全文
相关推荐
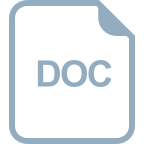
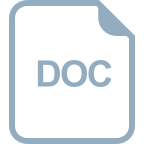
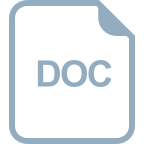
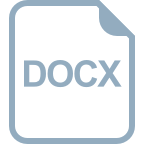
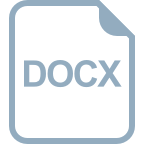
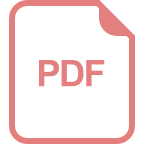
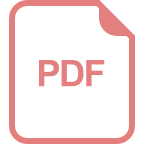
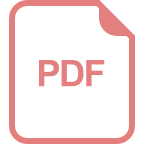
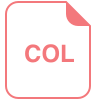
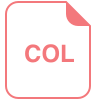
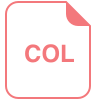





