def get_binary_img(timg): bin_img = np.zeros(shape=timg.shape, dtype=np.uint8) h = timg.shape[0] # 表示y的大小 w = timg.shape[1] # 表示x的大小 # print(" h = {} w = {}".format(h,w)) for i in range(h): # 从上到下 for j in range(w): bin_img[i][j] = 255 if timg[i][j] > 200 else 0 return bin_img
时间: 2024-03-18 20:43:16 浏览: 175
这段代码实现了一个简单的图像二值化函数`get_binary_img`,其输入参数`timg`为一张灰度图像。函数返回一个二值化后的图像`bin_img`,其中像素值为0或255。
具体实现为,先创建一个与输入图像`timg`具有相同形状和数据类型的全零数组`bin_img`,然后遍历输入图像的每个像素,将其灰度值与阈值200进行比较,如果大于阈值则将对应的二值图像像素置为255,否则置为0。最后返回二值化后的图像`bin_img`。
相关问题
import cv2 import matplotlib.pyplot as plt import numpy as np from skimage.measure import label, regionprops file_url = './data/origin/DJI_0081.jpg' output_url = './DJI_0081_ROI.jpg' def show_img(img, title): cv2.namedWindow(title, cv2.WINDOW_NORMAL) cv2.imshow(title, img) def output_img(img, url): cv2.imwrite(url, img, [int(cv2.IMWRITE_PNG_COMPRESSION), 9]) # 使用2g-r-b分离 src = cv2.imread(file_url) show_img(src, 'src') # 转换为浮点数进行计算 fsrc = np.array(src, dtype=np.float32) / 255.0 (b, g, r) = cv2.split(fsrc) gray = 2 * g - 0.9 * b - 1.1 * r # 求取最大值和最小值 (minVal, maxVal, minLoc, maxLoc) = cv2.minMaxLoc(gray) # 转换为u8类型,进行otsu二值化 gray_u8 = np.array((gray - minVal) / (maxVal - minVal) * 255, dtype=np.uint8) (thresh, bin_img) = cv2.threshold(gray_u8, -1.0, 255, cv2.THRESH_OTSU) show_img(bin_img, 'bin_img') def find_max_connected_component(binary_img): # 输出二值图像中所有的连通域 img_label, num = label(binary_img, connectivity=1, background=0, return_num=True) # connectivity=1--4 connectivity=2--8 # print('+++', num, img_label) # 输出连通域的属性,包括面积等 props = regionprops(img_label) resMatrix = np.zeros(img_label.shape).astype(np.uint8) # 只保留最大的连通域 max_area = 0 max_index = 0 for i in range(0, len(props)): if props[i].area > max_area: max_area = props[i].area max_index = i tmp = (img_label == max_index + 1).astype(np.uint8) resMatrix += tmp resMatrix *= 255 return resMatrix bin_img = find_max_connected_component(bin_img) show_img(bin_img, 'bin_img') # 得到彩色的图像 (b8, g8, r8) = cv2.split(src) color_img = cv2.merge([b8 & bin_img, g8 & bin_img, r8 & bin_img]) output_img(color_img, output_url) show_img(color_img, 'color_img') cv2.waitKey() cv2.destroyAllWindows()
这段代码是用来对一张图片进行处理,找到图片中最大的连通域,并将其保留下来。具体的处理过程如下:
1.读入图片并显示;
2.将图片转换成浮点数类型,方便做计算;
3.使用2g-r-b分离,得到灰度图像;
4.使用minMaxLoc函数找到灰度图像中的最大值和最小值;
5.将灰度图像转换成u8类型,方便做Otsu二值化;
6.使用Otsu二值化将图像进行二值化处理;
7.找到图像中最大的连通域;
8.将连通域保留下来,并输出彩色图像。
需要注意的是,代码中使用了一些第三方库,比如OpenCV和skimage,需要提前安装好。另外,代码中也有一些常量的设置,比如阈值等,可以根据具体需求进行调整。
改正此代码import cv2 import numpy as np import matplotlib.pyplot as plt image1 = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.12.tif') img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.12.tif',0) def bit_plane_slicing(src,high): height, width,high = src.shape dst = np.zeros((height, width), np.uint8) for i in range(0, height): for j in range(0, width): dst= plt.dec2bin(src[i,j],8) for high = 1 : 8: if str(high) == '0': dst(x, y, 9 - high) = 0; else: dst(x, y, 9 - high) = 1; return dst image2 = bit_plane_slicing(img,1) image3 = bit_plane_slicing(img, 2) plt.figure(figsize=(100,100)) plt.subplot(131) plt.imshow(image1,cmap='gray') plt.axis('off') plt.subplot(132) plt.imshow(image2,cmap='gray') plt.axis('off') plt.subplot(133) plt.imshow(image3,cmap='gray') plt.axis('off') plt.show()
import cv2
import numpy as np
import matplotlib.pyplot as plt
image1 = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.12.tif')
img = cv2.imread(r'E:\\postgraduate\\three\\DIP3E_Original_Images_CH03\\3.12.tif',0)
def bit_plane_slicing(src,high):
height, width = src.shape
dst = np.zeros((height, width, 8), np.uint8)
for i in range(0, height):
for j in range(0, width):
binary = format(src[i,j], '08b')
for bit in range(0, 8):
dst[i, j, bit] = binary[bit]
return dst[:, :, high]
image2 = bit_plane_slicing(img, 1)
image3 = bit_plane_slicing(img, 2)
plt.figure(figsize=(10,10))
plt.subplot(131)
plt.imshow(image1,cmap='gray')
plt.axis('off')
plt.subplot(132)
plt.imshow(image2,cmap='gray')
plt.axis('off')
plt.subplot(133)
plt.imshow(image3,cmap='gray')
plt.axis('off')
plt.show()
阅读全文
相关推荐
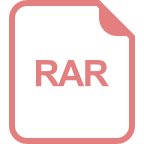
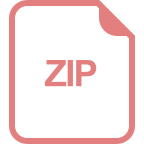




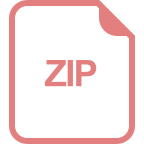
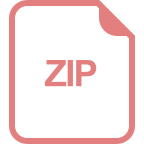
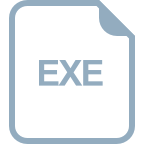
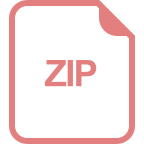
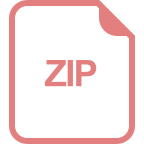
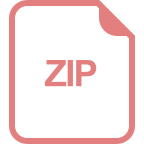
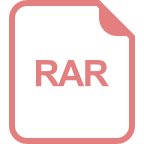
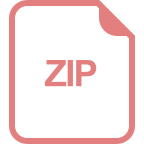
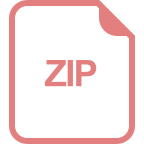
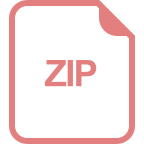
