C语言串口通信
时间: 2023-07-24 20:48:24 浏览: 138
C语言串口通信可以通过操作串口的设备文件来实现。以下是一个简单的例子,实现了从串口读取数据和向串口发送数据的基本操作。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main(int argc, char *argv[])
{
int fd;
struct termios options;
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
exit(1);
}
// 设置串口属性
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL | CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 从串口读取数据
char buf[255];
int n = read(fd, buf, sizeof(buf));
if (n > 0) {
buf[n] = '\0';
printf("Received: %s\n", buf);
}
// 向串口发送数据
const char *msg = "Hello, world!";
n = write(fd, msg, strlen(msg));
if (n < 0) {
perror("write");
exit(1);
}
// 关闭串口设备文件
close(fd);
return 0;
}
```
这段代码中,我们使用了 Linux 系统里的串口设备文件 `/dev/ttyS0`,也可以根据实际情况选择其他串口设备文件。通过 `open` 函数打开设备文件,并设置了一些串口属性,如波特率、数据位、停止位、校验位等。然后,我们可以使用 `read` 函数从串口读取数据,使用 `write` 函数向串口发送数据。最后,使用 `close` 函数关闭设备文件。
需要注意的是,串口通信可能涉及到硬件流控和软件流控等问题,具体实现方式要根据实际情况进行调整。
阅读全文
相关推荐
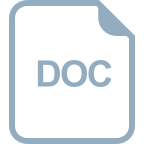
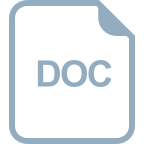
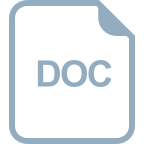
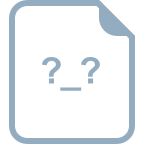
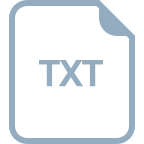
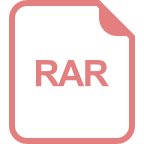
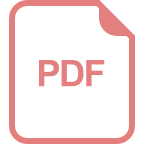
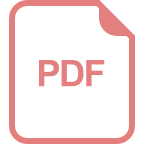
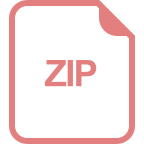
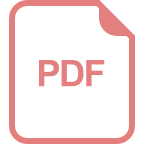
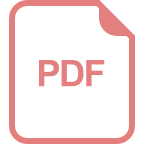
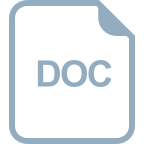
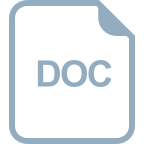
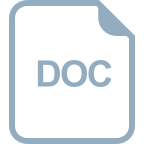
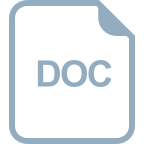
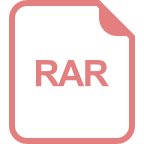
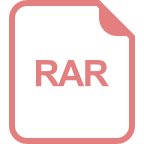