def call(self, x): x = self.fc1(x) x = self.fc2(x)
时间: 2024-04-11 08:12:20 浏览: 196
x = self.fc3(x)
return x
This is a code snippet for a neural network model's forward pass. The input 'x' is passed through three fully connected layers (fc1, fc2, and fc3) and the final output is returned. The 'call' function is typically used during training and inference to propagate the input through the model and obtain the output predictions.
相关问题
用tensorflow的layers.Layer模块改写class ChannelAttention(nn.Module): def init(self, in_planes, ratio = 4): super(ChannelAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool2d(1) self.max_pool = nn.AdaptiveMaxPool2d(1) self.fc1 = nn.Conv2d(in_planes, in_planes // ratio, 1, bias=False) self.relu1 = nn.ReLU() self.fc2 = nn.Conv2d(in_planes // ratio, in_planes, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x)))) max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x)))) out = avg_out + max_out return self.sigmoid(out)
以下是使用tensorflow的layers.Layer模块改写的ChannelAttention类:
```
import tensorflow as tf
from tensorflow.keras import layers
class ChannelAttention(layers.Layer):
def __init__(self, in_planes, ratio=4):
super(ChannelAttention, self).__init__()
self.avg_pool = layers.GlobalAveragePooling2D()
self.max_pool = layers.GlobalMaxPooling2D()
self.fc1 = layers.Conv2D(in_planes // ratio, 1, activation='relu', use_bias=False)
self.fc2 = layers.Conv2D(in_planes, 1, use_bias=False)
self.sigmoid = layers.Activation('sigmoid')
def call(self, x):
avg_out = self.fc2(self.fc1(self.avg_pool(x)))
max_out = self.fc2(self.fc1(self.max_pool(x)))
out = layers.add([avg_out, max_out])
return self.sigmoid(out)
```
在这个改写版本中,我使用了tensorflow的layers.Layer模块,并且对应用到了tensorflow中的层。使用layers.GlobalAveragePooling2D()代替了nn.AdaptiveAvgPool2d(1),使用layers.GlobalMaxPooling2D()代替了nn.AdaptiveMaxPool2d(1)。同时,我使用了layers.Conv2D()代替了nn.Conv2d(),并且在第一个卷积层中加入了激活函数。最后,我使用了layers.add()代替了avg_out + max_out。
class ChannelAttention(nn.Module): def __init__(self, in_planes, ratio = 4): super(ChannelAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool2d(1) self.max_pool = nn.AdaptiveMaxPool2d(1) self.fc1 = nn.Conv2d(in_planes, in_planes // ratio, 1, bias=False) self.relu1 = nn.ReLU() self.fc2 = nn.Conv2d(in_planes // ratio, in_planes, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x)))) max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x)))) out = avg_out + max_out return self.sigmoid(out) 改为tensorflow形式
在 TensorFlow 中,可以使用以下代码来实现相同的通道注意力模块:
```python
import tensorflow as tf
class ChannelAttention(tf.keras.layers.Layer):
def __init__(self, in_planes, ratio=4):
super(ChannelAttention, self).__init__()
self.avg_pool = tf.keras.layers.GlobalAvgPool2D()
self.max_pool = tf.keras.layers.GlobalMaxPool2D()
self.fc1 = tf.keras.layers.Conv2D(in_planes // ratio, kernel_size=1, strides=1, use_bias=False)
self.relu1 = tf.keras.layers.ReLU()
self.fc2 = tf.keras.layers.Conv2D(in_planes, kernel_size=1, strides=1, use_bias=False)
self.sigmoid = tf.keras.layers.Activation('sigmoid')
def call(self, x):
avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x))))
max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x))))
out = avg_out + max_out
return self.sigmoid(out)
```
在 TensorFlow 中,我们使用 `tf.keras.layers` 模块来定义网络层,使用 `call()` 方法来实现前向传播。这里的 `GlobalAvgPool2D` 和 `GlobalMaxPool2D` 分别代替了 PyTorch 中的 `AdaptiveAvgPool2d` 和 `AdaptiveMaxPool2d`,它们也可以用来对不同尺寸的输入进行平均池化和最大池化。另外,TensorFlow 中没有 `nn.ReLU` 模块,我们需要使用 `tf.keras.layers.ReLU()` 来替代。其他的代码实现和 PyTorch 版本相同。
阅读全文
相关推荐
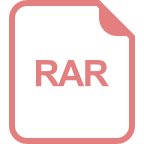









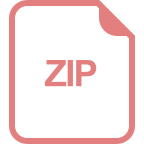