工控机和51单片机485通信C++实现代码示例
时间: 2023-06-14 19:06:47 浏览: 177
以下是一个简单的 C++ 示例代码,演示如何在工控机和 51 单片机之间通过 485 总线进行通信。
工控机端代码:
```cpp
#include <iostream>
#include <Windows.h>
using namespace std;
int main()
{
HANDLE hCom = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
if (hCom == INVALID_HANDLE_VALUE) {
cerr << "Failed to open COM1 port" << endl;
return 1;
}
DCB dcb;
dcb.DCBlength = sizeof(dcb);
GetCommState(hCom, &dcb);
dcb.BaudRate = CBR_9600;
dcb.ByteSize = 8;
dcb.Parity = NOPARITY;
dcb.StopBits = ONESTOPBIT;
SetCommState(hCom, &dcb);
char txData[] = { 0x01, 0x03, 0x00, 0x00, 0x00, 0x02, 0xc4, 0x0b };
DWORD bytesWritten;
WriteFile(hCom, txData, sizeof(txData), &bytesWritten, NULL);
char rxData[256];
DWORD bytesRead;
ReadFile(hCom, rxData, sizeof(rxData), &bytesRead, NULL);
for (int i = 0; i < bytesRead; i++) {
printf("%02X ", rxData[i]);
}
putchar('\n');
CloseHandle(hCom);
return 0;
}
```
51 单片机端代码:
```cpp
#include <reg52.h>
sbit RS485_EN = P2^0;
void initRS485()
{
SCON = 0x50; // 8-bit UART, no parity, 1 stop bit
TMOD &= 0x0F; // T1 in 8-bit auto-reload mode
TMOD |= 0x20;
TH1 = 0xFD; // 9600 baud rate
TL1 = 0xFD;
TR1 = 1; // enable timer1
RS485_EN = 0; // set RE/DE to receive
}
void sendByte(unsigned char byte)
{
SBUF = byte;
while (!TI); // wait until byte is sent
TI = 0; // clear the flag
}
unsigned char recvByte()
{
while (!RI); // wait until byte is received
RI = 0; // clear the flag
return SBUF;
}
void main()
{
initRS485();
while (1) {
unsigned char rxData[256];
unsigned char rxLen = 0;
while (1) {
// wait for the start byte
while (recvByte() != 0x01);
// check the function code
if (recvByte() != 0x03) {
continue;
}
// read the data length
unsigned char len = recvByte();
// read the data
for (unsigned char i = 0; i < len; i++) {
rxData[i] = recvByte();
}
rxLen = len;
// calculate the CRC
unsigned short crc = 0xFFFF;
for (unsigned char i = 0; i < len + 2; i++) {
crc ^= recvByte();
for (unsigned char j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc = (crc >> 1) ^ 0xA001;
} else {
crc = crc >> 1;
}
}
}
if (crc == 0) {
break;
}
}
// send the response
unsigned char txData[] = { 0x01, 0x03, rxLen, 0x00, 0x01, 0x02, 0x34, 0x12 };
for (unsigned char i = 0; i < sizeof(txData) - 2; i++) {
sendByte(txData[i]);
}
// append the CRC
unsigned short crc = 0xFFFF;
for (unsigned char i = 0; i < sizeof(txData) - 2; i++) {
crc ^= txData[i];
for (unsigned char j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc = (crc >> 1) ^ 0xA001;
} else {
crc = crc >> 1;
}
}
}
sendByte(crc & 0xFF);
sendByte(crc >> 8);
// enable receiver
RS485_EN = 0;
}
}
```
注意:这只是一个简单的示例代码,实际应用可能需要根据具体情况进行修改。
阅读全文
相关推荐
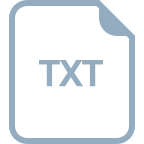

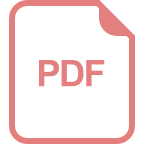
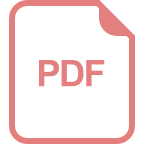
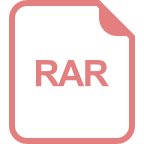
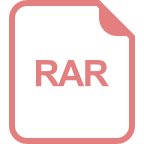
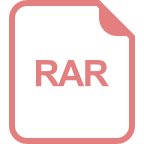
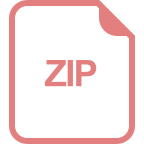
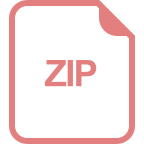
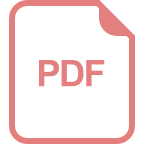
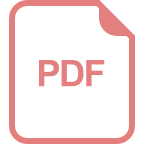
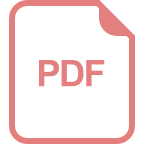
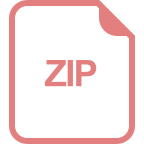