用C++写一个线程安全的线程池
时间: 2023-12-08 07:04:31 浏览: 56
一个线程池是由一组工作线程组成的,线程池中的线程可用于执行多个任务,这样可以减少线程创建和销毁的开销,提高程序的性能。下面是一个用C++编写的线程安全的线程池的代码示例:
```cpp
#include <iostream>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(size_t num_threads): stop(false) {
for (size_t i = 0; i < num_threads; ++i) {
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
}
template<class F>
void enqueue(F&& f) {
{
std::unique_lock<std::mutex> lock(queue_mutex);
tasks.emplace(std::forward<F>(f));
}
condition.notify_one();
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread &worker : workers)
worker.join();
}
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop;
};
```
在这个示例中,我们定义了一个ThreadPool类,它包含了线程池的核心功能。构造函数ThreadPool()用于创建一组工作线程,enqueue()函数用于将任务加入到线程池中,而析构函数~ThreadPool()用于停止线程池中的所有工作线程。
具体实现中,我们用一个std::queue<std::function<void()>>类型的tasks队列来存储所有加入的任务。由于线程池是多线程并发执行的,因此需要使用一个std::mutex类型的queue_mutex来保证多个线程同时操作任务队列时的线程安全性。当一个任务被加入到任务队列后,我们需要使用std::condition_variable类型的condition来通知工作线程有新的任务到达。
在工作线程中,我们使用了一个死循环来不断地获取任务并执行。在获取任务的过程中,我们需要使用std::unique_lock<std::mutex>类型的lock来保证线程安全性。当线程池被析构时,我们需要通知所有工作线程停止运行,并使用std::thread::join()函数等待工作线程退出。
相关推荐
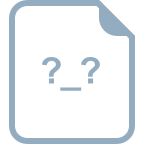














