delay and sum python
时间: 2024-01-05 11:46:24 浏览: 78
"Delay and sum" is a signal processing technique used in beamforming to combine signals received from multiple microphones or sensors. The basic idea is to introduce delays to the signals received by each microphone/sensor so that they arrive at a common point in space in phase, and then sum up the delayed signals to form a beam that is directional and focused.
Here's a simple implementation of delay and sum in Python:
```python
import numpy as np
def delay_and_sum(signals, delays):
"""
Combine signals using delay-and-sum beamforming.
signals: array of shape (num_mics, num_samples)
Array of signals received by each microphone/sensor.
delays: array of shape (num_mics,)
Array of delays to be applied to each signal, in samples.
Returns:
--------
combined_signal: array of shape (num_samples,)
Array of combined signal after delay-and-sum beamforming.
"""
num_mics, num_samples = signals.shape
max_delay = np.max(delays)
padded_signals = np.pad(signals, ((0, 0), (max_delay, 0)), mode='constant')
combined_signal = np.zeros(num_samples)
for i in range(num_samples):
delayed_signals = np.zeros((num_mics,))
for j in range(num_mics):
delayed_signals[j] = padded_signals[j, i+delays[j]]
combined_signal[i] = np.sum(delayed_signals)
return combined_signal
```
In this implementation, the `signals` input is an array of shape `(num_mics, num_samples)`, where `num_mics` is the number of microphones/sensors and `num_samples` is the number of samples in each signal. The `delays` input is an array of shape `(num_mics,)`, which specifies the delays to be applied to each signal, in samples.
The implementation pads the signals with zeros to accommodate the maximum delay, and then loops over each sample in the signals to apply the delays and sum up the delayed signals. The output is a combined signal of shape `(num_samples,)`.
Note that this is a basic implementation and there are many ways to optimize and improve it depending on the specific application.
阅读全文
相关推荐
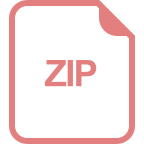
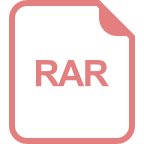




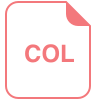
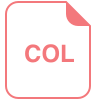
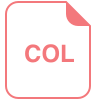
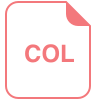








