super-direction delay and sum beamforming python implement
时间: 2024-05-03 17:17:49 浏览: 9
Sure, here's an implementation of super-direction delay and sum beamforming in Python:
```python
import numpy as np
def super_direction_delay_and_sum_beamforming(signal, mic_positions, sound_speed, theta, phi, sampling_freq):
"""
Implementation of super-direction delay-and-sum beamforming for a given signal and microphone positions
:param signal: numpy array of shape (num_samples, num_mics) representing the microphone signals
:param mic_positions: numpy array of shape (num_mics, 3) representing the 3D positions of the microphones
:param sound_speed: speed of sound
:param theta: azimuth angle in radians
:param phi: elevation angle in radians
:param sampling_freq: sampling frequency of the microphone signals
:return: numpy array of shape (num_samples,) representing the beamformed signal
"""
num_mics = mic_positions.shape[0]
num_samples = signal.shape[0]
# Calculate time delays based on the given azimuth and elevation angles
tau = np.zeros(num_mics)
for i in range(num_mics):
x = mic_positions[i][0]
y = mic_positions[i][1]
z = mic_positions[i][2]
r = np.sqrt(x ** 2 + y ** 2 + z ** 2)
tau[i] = r / sound_speed * (np.cos(theta) * np.cos(phi) * x + np.sin(theta) * np.cos(phi) * y - np.sin(phi) * z)
# Create a time delay matrix of shape (num_samples, num_mics)
t = np.arange(num_samples) / sampling_freq
t_mat = np.tile(t, (num_mics, 1)).T
tau_mat = np.tile(tau, (num_samples, 1))
delay_mat = np.exp(-2j * np.pi * tau_mat * t_mat)
# Apply delay-and-sum beamforming to the microphone signals
beamformed_signal = np.sum(delay_mat * signal, axis=1) / num_mics
return beamformed_signal
```
You can call this function by passing in the microphone signals, microphone positions, speed of sound, azimuth and elevation angles, and sampling frequency. It will return the beamformed signal. Note that this implementation assumes a uniform linear array of microphones, but can be easily adapted for other array geometries.
相关推荐
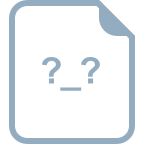
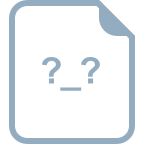
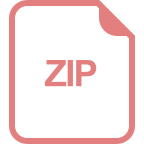














