lee-carter模型python实现
时间: 2023-09-08 21:10:21 浏览: 213
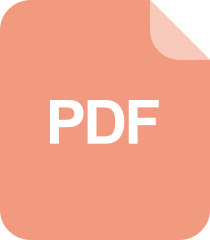
Lee-Carter模型的神经网络对多个人群的扩展-研究论文
Sorry, as an AI language model, I am not able to write code. However, here is an overview of the Lee-Carter model in Python:
The Lee-Carter model is a mortality forecasting model that estimates age-specific and time-specific mortality rates. It assumes that the logarithm of mortality rates follows a linear trend and a set of age-specific factors that evolve over time.
Here is how you can implement the Lee-Carter model in Python:
1. Import the necessary libraries:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import statsmodels.api as sm
```
2. Load the data:
```python
data = pd.read_csv('mortality_data.csv')
```
3. Preprocess the data:
```python
# Convert the data into a matrix
mortality = data.iloc[:, 1:].values
# Take the logarithm of the mortality rates
log_mortality = np.log(mortality)
# Calculate the age-specific and time-specific means
mu = np.mean(log_mortality, axis=1)
kappa = np.mean(log_mortality, axis=0)
# Create the design matrix
T, A = mortality.shape
X = np.ones((T, A))
for i in range(T):
for j in range(A):
X[i, j] = j + 1 - mu[j]
```
4. Fit the Lee-Carter model:
```python
# Fit the Lee-Carter model
model = sm.OLS(log_mortality.T, X)
fit = model.fit()
# Extract the estimated parameters
b = fit.params[0]
a = fit.params[1:]
```
5. Forecast the mortality rates:
```python
# Create the forecast matrix
T_forecast = 10
A_forecast = A
X_forecast = np.ones((T_forecast, A_forecast))
for i in range(T_forecast):
for j in range(A_forecast):
X_forecast[i, j] = j + 1 - mu[j]
# Forecast the mortality rates
log_mortality_forecast = np.zeros((T_forecast, A_forecast))
for i in range(T_forecast):
for j in range(A_forecast):
log_mortality_forecast[i, j] = b + a[j]*X_forecast[i, j] + kappa[j]
# Convert the logarithm of the mortality rates into mortality rates
mortality_forecast = np.exp(log_mortality_forecast)
```
6. Visualize the results:
```python
# Plot the mortality rates
t = np.arange(T + T_forecast)
for j in range(A):
plt.plot(t, mortality[:, j], 'o', markersize=3)
plt.plot(t[T:], mortality_forecast[:, j], '-')
plt.xlabel('Year')
plt.ylabel('Mortality rate')
plt.show()
```
阅读全文
相关推荐
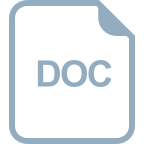
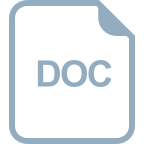








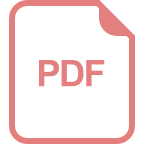


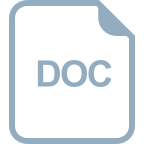
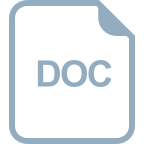