用Python实现smote算法
时间: 2023-08-31 08:28:58 浏览: 104
### 回答1:
我可以提供一个简单的Python实现SMOTE算法的例子:from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) x_res, y_res = sm.fit_resample(x, y)
### 回答2:
SMOTE(Synthetic Minority Over-sampling Technique)是一种数据增强算法,用于解决非平衡分类问题。它通过合成新的少数类样本来平衡数据集,从而改善分类器性能。
要实现SMOTE算法,可以使用Python编程语言,并按照以下步骤:
1. 导入必要的库,包括numpy和random。
2. 定义一个函数,用于计算两个样本之间的欧氏距离。
3. 定义一个主函数,输入参数包括原始不平衡的数据集、少数类样本的数量、以及需要合成的新样本数。
4. 根据原始数据集中的类别,将数据分成少数类和多数类两个集合。
5. 对于每个少数类样本,计算其与最近邻样本之间的欧氏距离。
6. 对于每个少数类样本,从其最近邻样本中随机选择一个样本。
7. 针对选择的样本,计算其与少数类样本之间的差值。
8. 随机生成一个0到1之间的比例因子。
9. 使用比例因子乘以差值,将其添加到少数类样本上,生成新的合成样本。
10. 将新样本添加到原始数据集中。
11. 重复步骤7到10,直到生成足够数量的新样本。
12. 返回合成后的数据集。
以上是使用Python实现SMOTE算法的大致步骤,具体的代码实现细节可以根据自己的需求进行调整和完善。
### 回答3:
SMOTE(Synthetic Minority Over-sampling Technique)是一种用于处理分类问题中严重不平衡数据集的一种常用方法。它通过合成少数类样本来增加其在数据集中的比例,以便更好地训练机器学习模型。
要使用Python实现SMOTE算法,可以按以下步骤进行:
1. 导入所需的库和模块,例如numpy和sklearn等。
2. 加载原始数据集,并将其划分为少数类和多数类样本。
3. 计算少数类样本与其最近邻样本之间的欧氏距离。
4. 为每个少数类样本选择k个最近邻样本。
5. 对于每个少数类样本,计算合成样本。假设要合成N个新样本,可以通过在少数类样本和其k个最近邻样本之间按比例插值得到新样本。
6. 将合成样本添加到原始数据集中的少数类样本中。
7. 可以选择重复步骤3到6多次,以产生更多的合成样本。
8. 将新生成的数据集用于训练机器学习模型,例如使用逻辑回归或支持向量机等算法。
这只是一个大致的框架,具体的实现细节可能有所不同。在实际应用中,可以根据具体情况对算法进行优化和调整。
总而言之,通过使用Python编写代码,并根据以上步骤实现SMOTE算法,可以有效地处理分类问题中的不平衡数据集。
相关推荐
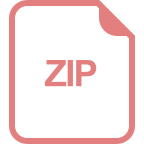
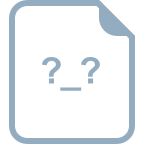

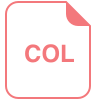











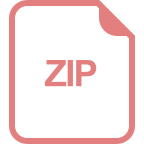