用c++面向对象写小游戏打飞机
时间: 2023-08-13 10:51:35 浏览: 52
好的,我可以为您提供一个基于C++面向对象的小游戏打飞机的示例代码。以下是一个简单的实现方案:
```c++
#include <iostream>
#include <vector>
#include <time.h>
#include <conio.h>
#include <windows.h>
using namespace std;
const int WIDTH = 50; // 游戏窗口宽度
const int HEIGHT = 20; // 游戏窗口高度
const int ENEMY_NUM = 5; // 敌机数量
const int BULLET_NUM = 3; // 子弹数量
class GameObject
{
public:
int x, y; // 物体的坐标
char shape; // 物体的形状
GameObject(int _x, int _y, char _shape)
{
x = _x;
y = _y;
shape = _shape;
}
virtual void show() = 0; // 显示物体
virtual void update() = 0; // 更新物体
virtual bool isCollide(GameObject& other) = 0; // 判断是否碰撞
};
class Plane : public GameObject
{
public:
int life; // 飞机的生命值
int speed; // 飞机的速度
Plane(int _x, int _y, int _life, int _speed, char _shape)
: GameObject(_x, _y, _shape), life(_life), speed(_speed)
{}
void show() override
{
cout << shape;
}
void update() override
{
if (_kbhit()) // 检测是否有按键按下
{
char ch = _getch();
switch (ch)
{
case 'a': x--; break;
case 'd': x++; break;
case 'w': y--; break;
case 's': y++; break;
}
}
}
bool isCollide(GameObject& other) override
{
if (x == other.x && y == other.y)
{
life--;
return true;
}
return false;
}
};
class Enemy : public GameObject
{
public:
int speed; // 敌机的速度
Enemy(int _x, int _y, int _speed, char _shape)
: GameObject(_x, _y, _shape), speed(_speed)
{}
void show() override
{
cout << shape;
}
void update() override
{
y += speed;
if (y >= HEIGHT - 1) // 敌机飞出窗口,回到顶部
{
y = 0;
x = rand() % WIDTH;
}
}
bool isCollide(GameObject& other) override
{
if (x == other.x && y == other.y)
{
return true;
}
return false;
}
};
class Bullet : public GameObject
{
public:
int speed; // 子弹的速度
Bullet(int _x, int _y, int _speed, char _shape)
: GameObject(_x, _y, _shape), speed(_speed)
{}
void show() override
{
cout << shape;
}
void update() override
{
y -= speed;
if (y < 0) // 子弹飞出窗口,销毁
{
x = -1;
y = -1;
}
}
bool isCollide(GameObject& other) override
{
if (x == other.x && y == other.y)
{
x = -1;
y = -1;
return true;
}
return false;
}
};
class Game
{
public:
Plane* plane; // 玩家控制的飞机
vector<Enemy*> enemies; // 敌机列表
vector<Bullet*> bullets; // 子弹列表
Game()
{
srand(time(NULL));
plane = new Plane(WIDTH / 2, HEIGHT - 2, 3, 1, 'A');
// 创建敌机
for (int i = 0; i < ENEMY_NUM; i++)
{
int x = rand() % WIDTH;
int y = rand() % (HEIGHT / 2);
int speed = rand() % 2 + 1;
Enemy* enemy = new Enemy(x, y, speed, 'V');
enemies.push_back(enemy);
}
// 创建子弹
for (int i = 0; i < BULLET_NUM; i++)
{
Bullet* bullet = new Bullet(-1, -1, 2, '.');
bullets.push_back(bullet);
}
}
~Game()
{
delete plane;
for (int i = 0; i < enemies.size(); i++)
{
delete enemies[i];
}
for (int i = 0; i < bullets.size(); i++)
{
delete bullets[i];
}
}
void show()
{
system("cls"); // 清空控制台屏幕
// 显示飞机
plane->show();
// 显示敌机
for (int i = 0; i < enemies.size(); i++)
{
enemies[i]->show();
}
// 显示子弹
for (int i = 0; i < bullets.size(); i++)
{
bullets[i]->show();
}
// 显示分数和生命值
cout << endl;
cout << "Score: " << (ENEMY_NUM - enemies.size()) << endl;
cout << "Life: " << plane->life << endl;
}
void update()
{
// 更新飞机
plane->update();
// 更新敌机
for (int i = 0; i < enemies.size(); i++)
{
enemies[i]->update();
}
// 更新子弹
for (int i = 0; i < bullets.size(); i++)
{
bullets[i]->update();
}
// 处理碰撞
for (int i = 0; i < enemies.size(); i++)
{
if (plane->isCollide(*enemies[i])) // 玩家飞机与敌机碰撞
{
if (plane->life <= 0)
{
cout << "Game Over!" << endl;
exit(0);
}
}
for (int j = 0; j < bullets.size(); j++)
{
if (bullets[j]->isCollide(*enemies[i])) // 子弹与敌机碰撞
{
delete enemies[i];
enemies.erase(enemies.begin() + i);
break;
}
}
}
// 发射子弹
if (_kbhit())
{
char ch = _getch();
if (ch == ' ')
{
for (int i = 0; i < bullets.size(); i++)
{
if (bullets[i]->x == -1 && bullets[i]->y == -1)
{
bullets[i]->x = plane->x;
bullets[i]->y = plane->y - 1;
break;
}
}
}
}
Sleep(50); // 控制帧率
}
};
int main()
{
Game game;
while (true)
{
game.show();
game.update();
}
return 0;
}
```
这个小游戏打飞机是基于面向对象的思想实现的,其中定义了 `GameObject`、`Plane`、`Enemy` 和 `Bullet` 四个类,分别代表游戏中的游戏对象。`Plane` 类表示玩家控制的飞机,`Enemy` 类表示敌机,`Bullet` 类表示子弹。`GameObject` 类是这三个类的基类,抽象了游戏对象的公共属性和行为。
在 `Game` 类中,创建了一个玩家飞机、五架敌机和三个子弹的对象,并在 `update` 方法中处理游戏逻辑,包括更新游戏对象的位置、检测碰撞、发射子弹等操作。在 `show` 方法中,将游戏对象显示在控制台上,包括玩家飞机、敌机、子弹、分数和生命值。
在 `main` 函数中,创建了一个 `Game` 对象,并在一个无限循环中调用 `show` 和 `update` 方法,实现了游戏的运行。
当玩家飞机与敌机碰撞时,玩家飞机的生命值会减一,当生命值小于等于零时游戏结束。当子弹与敌机碰撞时,敌机会被销毁,并增加分数。玩家可以通过空格键发射子弹。
这只是一个简单的示例,您可以根据自己的需要对代码进行修改和扩展。
相关推荐
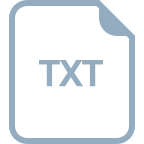
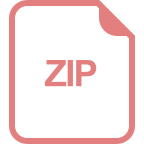
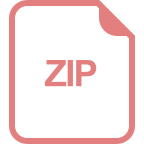














