对于一个包含自然数的列表nums,计算他的度
时间: 2023-10-18 22:16:43 浏览: 72
度是指列表中出现最多的元素的出现次数。
算法:
1. 遍历列表nums,用一个字典freq记录每个元素出现的次数。
2. 找到freq中出现次数最多的元素的出现次数max_freq。
3. 遍历freq,找到所有出现次数为max_freq的元素,将它们的出现次数累加起来得到度。
Python代码:
```python
def find_degree(nums):
freq = {}
for num in nums:
if num in freq:
freq[num] += 1
else:
freq[num] = 1
max_freq = max(freq.values())
degree = 0
for num, f in freq.items():
if f == max_freq:
degree += max_freq
return degree
```
示例:
```python
>>> find_degree([1, 2, 2, 3, 1])
4
>>> find_degree([1, 2, 3, 4, 5])
1
>>> find_degree([1, 2, 2, 3, 3, 3, 1, 1])
6
```
相关问题
对于一个包含自然数的列表nums,计算他的度
度是指列表中出现次数最多的元素的出现次数。
算法步骤:
1. 遍历列表nums,将每个元素出现的次数保存在一个字典中,字典的键为元素值,值为出现次数。
2. 找到字典中出现次数最多的值,即为列表的度。
3. 返回度。
Python代码实现:
```python
from collections import defaultdict
def find_degree(nums):
freq = defaultdict(int)
for num in nums:
freq[num] += 1
max_freq = max(freq.values())
degree = sum(1 for f in freq.values() if f == max_freq)
return degree
```
示例:
```python
>>> nums = [1, 2, 2, 3, 1, 4, 2]
>>> find_degree(nums)
3
```
解释:数字2在列表中出现了3次,是出现次数最多的元素,因此列表的度为3。
python 对于一个包含自然数的列表nums,计算他的度。
可以用字典来记录每个数字出现的次数,然后再遍历一遍字典找到出现次数最多的数字即可。
代码如下:
```python
from collections import defaultdict
def find_degree(nums):
count = defaultdict(int)
for num in nums:
count[num] += 1
max_count = max(count.values())
degree = 0
for key, value in count.items():
if value == max_count:
degree = max(degree, nums.count(key))
return degree
```
在这个代码中,我们先用defaultdict来创建一个默认值为0的字典count,然后遍历nums列表,统计每个数字出现的次数。接着,我们找到count字典中出现次数最多的数字的出现次数max_count。最后,我们再遍历一遍count字典,找到出现次数为max_count的数字中,最长子数组的长度degree。
阅读全文
相关推荐
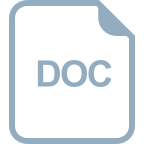
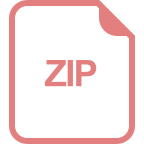












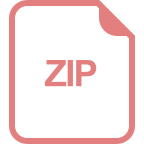
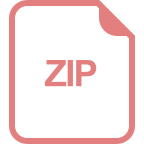