c++用类实现链表及增删改查
时间: 2023-10-10 15:06:12 浏览: 65
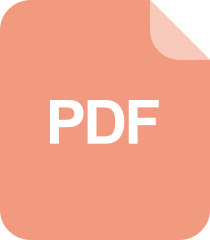
关于双向链表的增删改查和排序的C++实现
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int value) {
data = value;
next = nullptr;
}
};
class LinkedList {
private:
Node* head;
public:
LinkedList() {
head = nullptr;
}
void insert(int value) {
Node* newNode = new Node(value);
if (head == nullptr) {
head = newNode;
}
else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void remove(int value) {
if (head == nullptr) {
cout << "List is empty!" << endl;
}
else if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
}
else {
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next == nullptr) {
cout << "Value not found!" << endl;
}
else {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
}
void modify(int oldValue, int newValue) {
Node* current = head;
while (current != nullptr && current->data != oldValue) {
current = current->next;
}
if (current == nullptr) {
cout << "Value not found!" << endl;
}
else {
current->data = newValue;
}
}
void search(int value) {
Node* current = head;
while (current != nullptr && current->data != value) {
current = current->next;
}
if (current == nullptr) {
cout << "Value not found!" << endl;
}
else {
cout << "Value found!" << endl;
}
}
void display() {
if (head == nullptr) {
cout << "List is empty!" << endl;
}
else {
Node* current = head;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
}
};
int main() {
LinkedList list;
list.insert(5);
list.insert(10);
list.insert(15);
list.insert(20);
list.insert(25);
list.display();
list.remove(15);
list.display();
list.modify(10, 12);
list.display();
list.search(20);
list.search(30);
return 0;
}
阅读全文
相关推荐

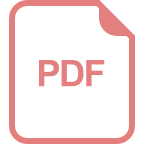
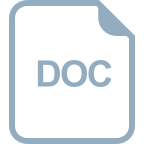
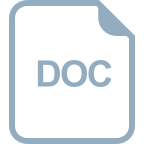



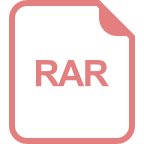
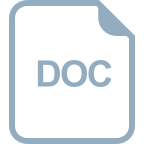
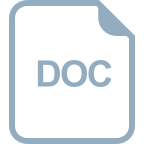
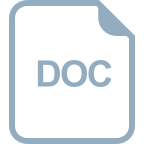



