#include <iostream> #include <vector> #include <algorithm> #include <string> using namespace std; // 定义一个基类Shape class Shape { public: virtual double getArea() = 0; // 纯虚函数 virtual string getName() = 0; // 纯虚函数 void setWidth(double w) { width = w; } void setHeight(double h) { height = h; } protected: double width; double height; }; // 定义派生类Rectangle class Rectangle: public Shape { public: double getArea() { return (width * height); } string getName() { return "矩形"; } }; // 定义派生类Triangle class Triangle: public Shape { public: double getArea() { return (width * height)/2; } string getName() { return "三角形"; } }; // 定义一个模板函数,用于计算图形集合的总面积 template<typename T> double getTotalArea(vector<T>& shapes) { double total = 0; for (typename vector<T>::iterator it = shapes.begin(); it != shapes.end();++it) { total += it ->getArea(); } return total; } // 定义一个函数对象,用于比较两个图形的面积大小 class CompareShapes { public: bool operator()(Shape* a, Shape* b) { return a->getArea() < b->getArea(); } }; int main() { vector<Shape*> shapes; Rectangle rect1; rect1.setWidth(5); rect1.setHeight(7); Rectangle rect2; rect2.setWidth(3); rect2.setHeight(4); Triangle tri1; tri1.setWidth(5); tri1.setHeight(7); Triangle tri2; tri2.setWidth(3); tri2.setHeight(4); shapes.push_back(&rect1); shapes.push_back(&rect2); shapes.push_back(&tri1); shapes.push_back(&tri2); // 输出图形集合的总面积 cout << "图形集合的总面积为:" << getTotalArea(shapes) << endl; // 对图形集合进行排序 sort(shapes.begin(), shapes.end(), CompareShapes()); // 输出图形集合中面积最大的图形的名称 cout << "图形集合中面积最大的图形是:" << shapes.back()->getName() << endl; return 0; }检查这段代码为什么出错
时间: 2024-01-14 07:04:06 浏览: 79
在代码开头的 `#include` 部分,每个头文件之间应该用换行符或分号隔开,而不是空格。修改后的代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
using namespace std;
// 定义一个基类Shape
class Shape {
public:
virtual double getArea() = 0; // 纯虚函数
virtual string getName() = 0; // 纯虚函数
void setWidth(double w) { width = w; }
void setHeight(double h) { height = h; }
protected:
double width;
double height;
};
// 定义派生类Rectangle
class Rectangle: public Shape {
public:
double getArea() {
return (width * height);
}
string getName() {
return "矩形";
}
};
// 定义派生类Triangle
class Triangle: public Shape {
public:
double getArea() {
return (width * height)/2;
}
string getName() {
return "三角形";
}
};
// 定义一个模板函数,用于计算图形集合的总面积
template<typename T>
double getTotalArea(vector<T>& shapes) {
double total = 0;
for (typename vector<T>::iterator it = shapes.begin(); it != shapes.end();++it) {
total += it ->getArea();
}
return total;
}
// 定义一个函数对象,用于比较两个图形的面积大小
class CompareShapes {
public:
bool operator()(Shape* a, Shape* b) {
return a->getArea() < b->getArea();
}
};
int main() {
vector<Shape*> shapes;
Rectangle rect1;
rect1.setWidth(5);
rect1.setHeight(7);
Rectangle rect2;
rect2.setWidth(3);
rect2.setHeight(4);
Triangle tri1;
tri1.setWidth(5);
tri1.setHeight(7);
Triangle tri2;
tri2.setWidth(3);
tri2.setHeight(4);
shapes.push_back(&rect1);
shapes.push_back(&rect2);
shapes.push_back(&tri1);
shapes.push_back(&tri2);
// 输出图形集合的总面积
cout << "图形集合的总面积为:" << getTotalArea(shapes) << endl;
// 对图形集合进行排序
sort(shapes.begin(), shapes.end(), CompareShapes());
// 输出图形集合中面积最大的图形的名称
cout << "图形集合中面积最大的图形是:" << shapes.back()->getName() << endl;
return 0;
}
```
另外,如果你的编译器出现了“no matching function for call to 'sort'” 的错误,可以添加一个比较函数的参数,修改 CompareShapes 类的定义如下:
```c++
class CompareShapes {
public:
bool operator()(Shape* a, Shape* b) {
return a->getArea() < b->getArea();
}
bool operator()(Shape a, Shape b) { // 添加这个比较函数
return a.getArea() < b.getArea();
}
};
```
然后在调用 `sort` 函数时,将比较函数改为 `CompareShapes()`,即可解决该错误。
阅读全文
相关推荐
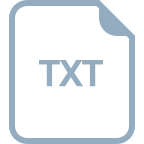
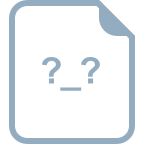
















