运用C++语言实现一个图书管理系统,需要代码注释,要描述书籍类、图书类型类、图书类。设计基类和派生类,并运用多态性和虚函数的知识。要求设计具有继承与派生以及多态性的类。 要实现的主要功能如下: 1)建立书籍类、图书类型类、图书类 2)书籍、图书类型、图书信息的初始化 3)书籍、图书类型、图书信息的添加、修改、删除、查找 4)图书信息的输出 5)对图书信息按编号、书名排序 6)将图书信息保存为文件 6)读入文件
时间: 2023-11-13 16:04:19 浏览: 174
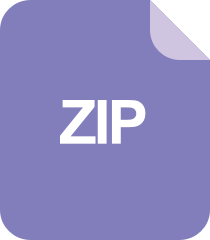
C/C++图书管理系统,支持添加图书,查找图书(自己录入),删除图书,借书还书。

很高兴能为您解答这个问题。以下是一个简单的图书管理系统的C++代码,注释描述了书籍类、图书类型类、图书类的设计,以及多态性和虚函数的使用。请注意,这只是一个简单的实现,可以根据需要进行修改和扩展。
```c++
#include<iostream>
#include<fstream>
#include<vector>
#include<algorithm>
using namespace std;
// 图书类型类
class BookType {
public:
BookType(string name = "", string description = "") {
this->name = name;
this->description = description;
}
string getName() const {
return name;
}
void setName(string name) {
this->name = name;
}
string getDescription() const {
return description;
}
void setDescription(string description) {
this->description = description;
}
private:
string name; // 图书类型名称
string description; // 图书类型描述
};
// 书籍类
class Book {
public:
Book(string id = "", string name = "", string author = "", double price = 0, BookType type = BookType()) {
this->id = id;
this->name = name;
this->author = author;
this->price = price;
this->type = type;
}
string getId() const {
return id;
}
void setId(string id) {
this->id = id;
}
string getName() const {
return name;
}
void setName(string name) {
this->name = name;
}
string getAuthor() const {
return author;
}
void setAuthor(string author) {
this->author = author;
}
double getPrice() const {
return price;
}
void setPrice(double price) {
this->price = price;
}
BookType getType() const {
return type;
}
void setType(BookType type) {
this->type = type;
}
virtual void printInfo() const { // 虚函数,用于输出图书信息
cout << "编号:" << id << endl;
cout << "名称:" << name << endl;
cout << "作者:" << author << endl;
cout << "价格:" << price << endl;
cout << "类型:" << type.getName() << endl;
}
private:
string id; // 书籍编号
string name; // 书籍名称
string author; // 书籍作者
double price; // 书籍价格
BookType type; // 书籍类型
};
// 普通图书类,继承自书籍类
class NormalBook : public Book {
public:
NormalBook(string id = "", string name = "", string author = "", double price = 0, BookType type = BookType()) : Book(id, name, author, price, type) {}
};
// 电子图书类,继承自书籍类
class EBook : public Book {
public:
EBook(string id = "", string name = "", string author = "", double price = 0, BookType type = BookType(), string format = "") : Book(id, name, author, price, type) {
this->format = format;
}
string getFormat() const {
return format;
}
void setFormat(string format) {
this->format = format;
}
virtual void printInfo() const { // 重载虚函数,用于输出电子图书信息
Book::printInfo();
cout << "格式:" << format << endl;
}
private:
string format; // 电子图书格式
};
// 图书管理类
class BookManager {
public:
void init() { // 初始化图书信息
BookType type1("计算机科学", "关于计算机科学的图书");
BookType type2("文学", "关于文学的图书");
books.push_back(new NormalBook("001", "C++ Primer", "Lippman", 65.0, type1));
books.push_back(new NormalBook("002", "Java核心技术", "Horstmann", 59.0, type1));
books.push_back(new EBook("003", "红楼梦", "曹雪芹", 29.0, type2, "PDF"));
books.push_back(new EBook("004", "西游记", "吴承恩", 39.0, type2, "EPUB"));
}
void addBook(Book* book) { // 添加图书
books.push_back(book);
}
void modifyBook(string id, Book* book) { // 修改图书
for (int i = 0; i < books.size(); i++) {
if (books[i]->getId() == id) {
books[i] = book;
break;
}
}
}
void deleteBook(string id) { // 删除图书
for (int i = 0; i < books.size(); i++) {
if (books[i]->getId() == id) {
books.erase(books.begin() + i);
break;
}
}
}
Book* findBookById(string id) const { // 按编号查找图书
for (int i = 0; i < books.size(); i++) {
if (books[i]->getId() == id) {
return books[i];
}
}
return NULL;
}
vector<Book*> findBookByName(string name) const { // 按名称查找图书
vector<Book*> result;
for (int i = 0; i < books.size(); i++) {
if (books[i]->getName() == name) {
result.push_back(books[i]);
}
}
return result;
}
void printAllBooks() const { // 输出所有图书信息
for (int i = 0; i < books.size(); i++) {
books[i]->printInfo();
}
}
void sortById() { // 按编号排序
sort(books.begin(), books.end(), [](Book* a, Book* b) { return a->getId() < b->getId(); });
}
void sortByName() { // 按名称排序
sort(books.begin(), books.end(), [](Book* a, Book* b) { return a->getName() < b->getName(); });
}
void saveToFile(string filename) const { // 保存到文件
ofstream ofs(filename);
if (ofs) {
for (int i = 0; i < books.size(); i++) {
ofs << books[i]->getId() << ",";
ofs << books[i]->getName() << ",";
ofs << books[i]->getAuthor() << ",";
ofs << books[i]->getPrice() << ",";
ofs << books[i]->getType().getName() << ",";
if (dynamic_cast<EBook*>(books[i])) { // 判断是否为电子图书
ofs << dynamic_cast<EBook*>(books[i])->getFormat() << endl;
}
else {
ofs << endl;
}
}
}
ofs.close();
}
void loadFromFile(string filename) { // 从文件读取
ifstream ifs(filename);
if (ifs) {
string line;
while (getline(ifs, line)) {
vector<string> fields;
string field;
for (int i = 0; i < line.length(); i++) {
if (line[i] == ',') {
fields.push_back(field);
field = "";
}
else {
field += line[i];
}
}
BookType type(fields[4], "");
if (fields.size() == 6) { // 电子图书
books.push_back(new EBook(fields[0], fields[1], fields[2], stod(fields[3]), type, fields[5]));
}
else { // 普通图书
books.push_back(new NormalBook(fields[0], fields[1], fields[2], stod(fields[3]), type));
}
}
}
ifs.close();
}
private:
vector<Book*> books; // 存储图书信息
};
int main() {
BookManager manager;
manager.init(); // 初始化图书信息
manager.printAllBooks(); // 输出所有图书信息
cout << endl;
// 添加图书
BookType type1("历史", "关于历史的图书");
Book* book1 = new NormalBook("005", "中国古代史", "王国维", 45.0, type1);
manager.addBook(book1);
// 修改图书
BookType type2("哲学", "关于哲学的图书");
Book* book2 = new NormalBook("002", "Java从入门到精通", "Horstmann", 69.0, type2);
manager.modifyBook("002", book2);
// 删除图书
manager.deleteBook("004");
// 按编号查找图书
Book* book3 = manager.findBookById("001");
if (book3 != NULL) {
book3->printInfo();
}
cout << endl;
// 按名称查找图书
vector<Book*> books = manager.findBookByName("Java从入门到精通");
for (int i = 0; i < books.size(); i++) {
books[i]->printInfo();
}
cout << endl;
// 按编号排序
manager.sortById();
manager.printAllBooks();
cout << endl;
// 按名称排序
manager.sortByName();
manager.printAllBooks();
cout << endl;
// 保存到文件
manager.saveToFile("books.txt");
// 从文件读取
BookManager manager2;
manager2.loadFromFile("books.txt");
manager2.printAllBooks();
return 0;
}
```
以上代码实现了一个简单的图书管理系统,包括了书籍类、图书类型类、图书类的设计,以及多态性和虚函数的使用。主要功能包括图书信息的初始化、添加、修改、删除、查找、排序、输出,以及将图书信息保存到文件和从文件读取。
阅读全文
相关推荐
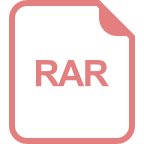
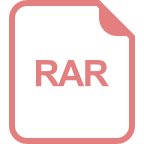











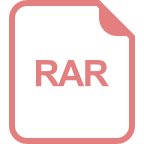
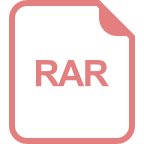
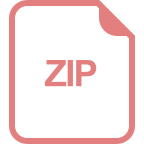