编写C++程序:如果用广度优先找最短路径,必须记录每个结点的父节点(parent),然后从终点沿parent向前搜索到起始结点.而不能够仅记录dist来找最短路径。
时间: 2024-02-11 16:10:03 浏览: 16
以下是一个简单的C++程序,用于使用广度优先搜索找到最短路径,并记录每个结点的父节点:
```c++
#include <iostream>
#include <queue>
#include <vector>
#include <cstring>
using namespace std;
const int MAXN = 100;
vector<int> G[MAXN]; // 图的邻接表表示
int dist[MAXN]; // 记录每个结点到起点的最短距离
int parent[MAXN]; // 记录每个结点的父节点
bool visited[MAXN]; // 记录每个结点是否已经访问
void bfs(int s) {
queue<int> q;
q.push(s);
visited[s] = true;
dist[s] = 0;
parent[s] = -1;
while (!q.empty()) {
int u = q.front();
q.pop();
for (int i = 0; i < G[u].size(); i++) {
int v = G[u][i];
if (!visited[v]) {
visited[v] = true;
dist[v] = dist[u] + 1;
parent[v] = u;
q.push(v);
}
}
}
}
int main() {
int n = 6; // 结点数目
int m = 7; // 边数目
memset(visited, false, sizeof(visited));
memset(dist, -1, sizeof(dist)); // 初始化距离为-1
memset(parent, -1, sizeof(parent)); // 初始化父节点为-1
// 添加边
G[1].push_back(2);
G[1].push_back(3);
G[2].push_back(4);
G[2].push_back(5);
G[3].push_back(5);
G[4].push_back(6);
G[5].push_back(6);
int start = 1; // 起点
int end = 6; // 终点
bfs(start);
if (visited[end]) {
cout << "The shortest distance from " << start << " to " << end << " is " << dist[end] << endl;
cout << "The path is: ";
for (int u = end; u != -1; u = parent[u]) {
cout << u << " ";
}
cout << endl;
} else {
cout << "No path found from " << start << " to " << end << endl;
}
return 0;
}
```
在这个程序中,我们使用了一个邻接表来表示图。对于每个结点,我们记录了它到起点的最短距离`dist`、它的父节点`parent`和它是否已经访问过`visited`。在广度优先搜索中,我们从起点开始搜索,依次遍历它的邻居结点,并记录它们的距离、父节点和是否已经访问。一旦找到了终点,我们就可以根据每个结点的父节点沿着最短路径回溯到起点,输出路径上的结点即可。
相关推荐
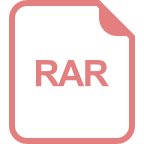
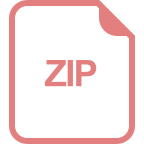














