python实现图像白平衡
时间: 2023-07-11 09:13:11 浏览: 52
图像白平衡是指对图像中的色温进行调整,以使图像中的白色区域看上去更加自然。以下是一种基于灰度世界假设的 Python 实现方法:
```python
import cv2
import numpy as np
def gray_world(img):
# 将图像转换为浮点数类型
img_float = img.astype(np.float32)
# 计算红、绿、蓝三个通道的平均值
avg_R = np.mean(img_float[:,:,2])
avg_G = np.mean(img_float[:,:,1])
avg_B = np.mean(img_float[:,:,0])
# 计算平均灰度值
avg_gray = (avg_R + avg_G + avg_B) / 3
# 计算红、绿、蓝三个通道的增益系数
Kr = avg_gray / avg_R
Kg = avg_gray / avg_G
Kb = avg_gray / avg_B
# 根据增益系数对图像进行调整
img_white_balance = np.zeros_like(img_float)
img_white_balance[:,:,2] = Kr * img_float[:,:,2]
img_white_balance[:,:,1] = Kg * img_float[:,:,1]
img_white_balance[:,:,0] = Kb * img_float[:,:,0]
# 将图像转换为 8 位无符号整数类型
img_white_balance = np.clip(img_white_balance, 0, 255).astype(np.uint8)
return img_white_balance
```
使用方法:
```python
img = cv2.imread('example.jpg')
img_white_balance = gray_world(img)
cv2.imshow('Original', img)
cv2.imshow('White balance', img_white_balance)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中 `example.jpg` 是待处理的图像文件名。运行结果将会显示原始图像和白平衡调整后的图像。
相关推荐
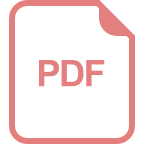
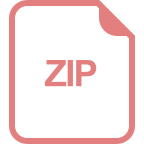














