用java语言写一个按模板纵向导出的Demo和算法
时间: 2023-05-18 21:05:32 浏览: 160
这里提供一个简单的Java程序,用于按照模板纵向导出数据。该程序使用Apache POI库来操作Excel文件。
算法:
1. 读取Excel模板文件
2. 创建新的Excel文件
3. 遍历模板文件中的每个单元格,将其内容复制到新的Excel文件中对应的单元格中
4. 将需要导出的数据按照模板中的格式填充到新的Excel文件中
5. 保存新的Excel文件
Java代码:
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelExportDemo {
public static void main(String[] args) {
String templateFilePath = "template.xlsx";
String outputFilePath = "output.xlsx";
Map<String, String> data = new HashMap<>();
data.put("name", "张三");
data.put("age", "20");
data.put("gender", "男");
data.put("address", "北京市朝阳区");
try {
Workbook templateWorkbook = new XSSFWorkbook(new FileInputStream(templateFilePath));
Workbook outputWorkbook = new XSSFWorkbook();
Sheet templateSheet = templateWorkbook.getSheetAt(0);
Sheet outputSheet = outputWorkbook.createSheet();
for (Row templateRow : templateSheet) {
Row outputRow = outputSheet.createRow(templateRow.getRowNum());
for (Cell templateCell : templateRow) {
Cell outputCell = outputRow.createCell(templateCell.getColumnIndex());
outputCell.setCellStyle(templateCell.getCellStyle());
switch (templateCell.getCellType()) {
case STRING:
outputCell.setCellValue(templateCell.getStringCellValue());
break;
case NUMERIC:
outputCell.setCellValue(templateCell.getNumericCellValue());
break;
case BOOLEAN:
outputCell.setCellValue(templateCell.getBooleanCellValue());
break;
case FORMULA:
outputCell.setCellFormula(templateCell.getCellFormula());
break;
default:
break;
}
}
}
for (String key : data.keySet()) {
Row outputRow = outputSheet.getRow(getRowIndexByKey(templateSheet, key));
Cell outputCell = outputRow.getCell(getColumnIndexByKey(templateSheet, key));
outputCell.setCellValue(data.get(key));
}
outputWorkbook.write(new FileOutputStream(outputFilePath));
outputWorkbook.close();
templateWorkbook.close();
System.out.println("导出成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
private static int getRowIndexByKey(Sheet sheet, String key) {
for (Row row : sheet) {
for (Cell cell : row) {
if (cell.getCellType() == CellType.STRING && cell.getStringCellValue().equals(key)) {
return row.getRowNum();
}
}
}
return -1;
}
private static int getColumnIndexByKey(Sheet sheet, String key) {
for (Row row : sheet) {
for (Cell cell : row) {
if (cell.getCellType() == CellType.STRING && cell.getStringCellValue().equals(key)) {
return cell.getColumnIndex();
}
}
}
return -1;
}
}
说明:
1. templateFilePath:Excel模板文件路径
2. outputFilePath:导出的Excel文件路径
3. data:需要导出的数据,以Map形式存储,key为模板中的占位符,value为实际数据
4. getRowIndexByKey:根据key获取行号
5. getColumnIndexByKey:根据key获取列号
阅读全文
相关推荐
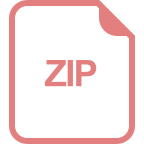


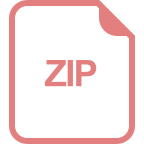
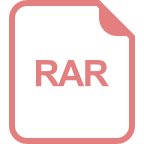
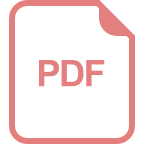
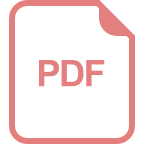
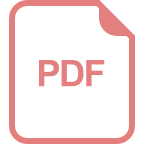
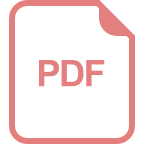
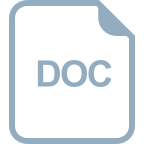
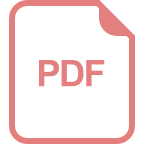
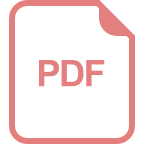
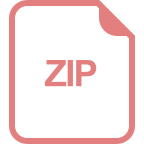
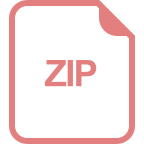
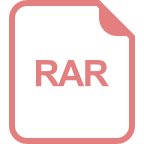
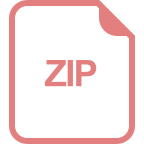