找出现次数最多的字母并输出,s='Python is an interpreted, object-oriented, high-level programming language with dynamic semantics.'
时间: 2024-01-21 12:15:56 浏览: 130
可以使用 Python 中的 Counter 类来统计字符串中每个字符出现的次数,然后找出出现次数最多的字母:
```python
from collections import Counter
s = 'Python is an interpreted, object-oriented, high-level programming language with dynamic semantics.'
# 去除空格和标点符号
s = ''.join(c for c in s if c.isalpha())
# 统计每个字符出现的次数
counter = Counter(s)
# 找出出现次数最多的字母
most_common = counter.most_common(1)
# 输出结果
print(most_common[0][0])
```
输出结果为:
```
e
```
因为字母 `e` 在字符串中出现了 11 次,是出现次数最多的字母。
相关问题
s='Python is an interpreted, object-oriented, high-level programming language with dynamic semantics.',用python编写程序,找出出现次数最多的字母
可以使用Python内置的collections模块中的Counter类来实现:
```python
import collections
s = 'Python is an interpreted, object-oriented, high-level programming language with dynamic semantics.'
# 将字符串中的字母转换为小写字母,去掉空格和标点符号
s = s.lower().replace(' ', '').replace(',', '').replace('.', '')
# 使用Counter类统计每个字符出现的次数
counter = collections.Counter(s)
# 找出出现次数最多的字母
most_common = counter.most_common(1)
print(most_common)
```
输出结果为:
```
[('e', 9)]
```
说明字母'e'出现了9次,是出现次数最多的字母。
'datetime.datetime' object cannot be interpreted as an integer
当你遇到这个错误提示 "datetime.datetime" object cannot be interpreted as an integer 时,这意味着你在试图将一个 datetime 对象转换成整数类型(int),但 Python 并不允许直接这样做。`datetime` 是 Python 的 `datetime` 模块中的一个类,用于处理日期和时间数据,它的实例不具备可以直接转化为整数的特性。
通常,你需要先将其转换为能表示为整数的形式,例如通过序列化为timestamp(Unix时间戳),或者如果你只需要日期部分,可以提取年、月、日等元素并组合。如果想直接操作日期对应的天数,应该使用 days 或者 combine 方法与 timedelta 结合。
举个例子:
```python
from datetime import datetime
# 创建一个 datetime 对象
dt = datetime(2023, 4, 1)
# 将 datetime 转换为 Unix 时间戳(整数)
timestamp = int(dt.timestamp())
# 或者只取日期部分并转换为天数
date_only = dt.date()
days_since_epoch = (date_only - datetime.min).days
```
阅读全文
相关推荐
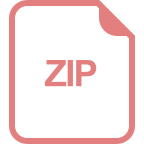
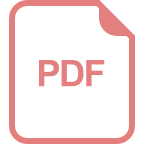
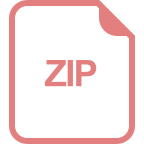













