icmp校验和计算代码
时间: 2023-05-15 11:05:21 浏览: 95
以下是一个简单的 ICMP 校验和计算代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <netinet/ip_icmp.h>
#include <netinet/ip.h>
unsigned short checksum(unsigned short *buf, int nwords)
{
unsigned long sum;
for (sum = 0; nwords > 0; nwords--)
sum += *buf++;
sum = (sum >> 16) + (sum & 0xffff);
sum += (sum >> 16);
return (unsigned short)(~sum);
}
int main(int argc, char *argv[])
{
struct icmphdr icmp_hdr;
struct iphdr ip_hdr;
char packet[IP_MAXPACKET];
memset(packet, 0, IP_MAXPACKET);
// 构造 ICMP 报文头
icmp_hdr.type = ICMP_ECHO;
icmp_hdr.code = 0;
icmp_hdr.checksum = 0;
icmp_hdr.un.echo.id = 1234;
icmp_hdr.un.echo.sequence = 1;
// 计算 ICMP 校验和
icmp_hdr.checksum = checksum((unsigned short *)&icmp_hdr, sizeof(icmp_hdr) / 2);
// 构造 IP 报文头
ip_hdr.version = 4;
ip_hdr.ihl = 5;
ip_hdr.tos = 0;
ip_hdr.tot_len = sizeof(struct iphdr) + sizeof(struct icmphdr);
ip_hdr.id = htons(54321);
ip_hdr.frag_off = 0;
ip_hdr.ttl = 255;
ip_hdr.protocol = IPPROTO_ICMP;
ip_hdr.check = 0;
ip_hdr.saddr = inet_addr("192.168.1.1");
ip_hdr.daddr = inet_addr("192.168.1.2");
// 将 ICMP 报文头和 IP 报文头拼接起来
memcpy(packet, &ip_hdr, sizeof(ip_hdr));
memcpy(packet + sizeof(ip_hdr), &icmp_hdr, sizeof(icmp_hdr));
// 发送 ICMP 报文
// ...
return 0;
}
```
注意:这只是一个简单的示例代码,实际应用中需要根据具体情况进行修改和完善。
相关推荐
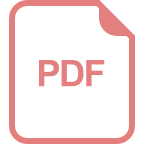
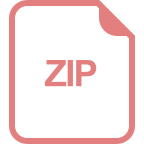














