icmp的校验和计算代码
时间: 2023-05-14 12:05:21 浏览: 285
以下是一个简单的 ICMP 校验和计算代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <netinet/ip_icmp.h>
#include <netinet/ip.h>
unsigned short checksum(unsigned short *ptr, int nbytes) {
register long sum;
unsigned short oddbyte;
register short answer;
sum = 0;
while (nbytes > 1) {
sum += *ptr++;
nbytes -= 2;
}
if (nbytes == 1) {
oddbyte = 0;
*((unsigned char *) &oddbyte) = *(unsigned char *) ptr;
sum += oddbyte;
}
sum = (sum >> 16) + (sum & 0xffff);
sum += (sum >> 16);
answer = (short) ~sum;
return answer;
}
int main() {
char buffer[1024];
memset(buffer, 0, sizeof(buffer));
struct iphdr *ip_header = (struct iphdr *) buffer;
struct icmphdr *icmp_header = (struct icmphdr *) (buffer + sizeof(struct iphdr));
ip_header->ihl = 5;
ip_header->version = 4;
ip_header->tos = 0;
ip_header->tot_len = sizeof(struct iphdr) + sizeof(struct icmphdr);
ip_header->id = htons(54321);
ip_header->frag_off = 0;
ip_header->ttl = 255;
ip_header->protocol = IPPROTO_ICMP;
ip_header->saddr = inet_addr("192.168.1.1");
ip_header->daddr = inet_addr("192.168.1.2");
icmp_header->type = ICMP_ECHO;
icmp_header->code = 0;
icmp_header->un.echo.id = 0;
icmp_header->un.echo.sequence = 0;
icmp_header->checksum = 0;
icmp_header->checksum = checksum((unsigned short *) icmp_header, sizeof(struct icmphdr));
printf("ICMP checksum: %d\n", icmp_header->checksum);
return 0;
}
```
这段代码演示了如何计算 ICMP 校验和。它首先构造了一个 IP 头和一个 ICMP 头,然后调用 `checksum` 函数计算 ICMP 校验和。
阅读全文
相关推荐
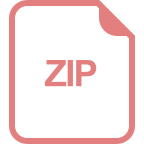
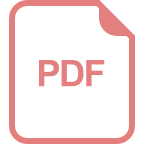








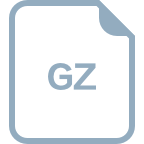
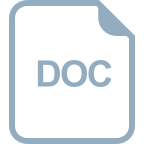




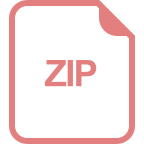