利用遗传算法和SVC模型进行特征工程筛选最优特征集的python代码实例
时间: 2023-08-10 12:08:18 浏览: 111
下面是一个利用遗传算法和SVC模型进行特征工程筛选最优特征集的Python代码实例:
```python
import numpy as np
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
# 加载数据集
cancer = load_breast_cancer()
X, y = cancer.data, cancer.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义适应度函数
def fitness(features):
X_train_selected = X_train[:, features]
X_test_selected = X_test[:, features]
clf = SVC()
clf.fit(X_train_selected, y_train)
score = clf.score(X_test_selected, y_test)
return score
# 遗传算法
def genetic_algorithm(size, gensize, retain, random_select, mutate):
population = []
for i in range(size):
chromosome = np.ones(gensize)
chromosome[:int(0.5*gensize)] = 0
np.random.shuffle(chromosome)
population.append(chromosome)
for i in range(100):
scores = []
for chromosome in population:
score = fitness(np.where(chromosome == 1)[0])
scores.append((score, chromosome))
scores.sort(reverse=True)
ranked_chromosomes = [x[1] for x in scores]
population = ranked_chromosomes[:retain]
while len(population) < size:
if np.random.rand() < random_select:
population.append(ranked_chromosomes[np.random.randint(0, retain)])
else:
chromosome1 = ranked_chromosomes[np.random.randint(0, retain)]
chromosome2 = ranked_chromosomes[np.random.randint(0, retain)]
new_chromosome = chromosome1.copy()
mask = np.random.rand(len(new_chromosome)) < 0.5
new_chromosome[mask] = chromosome2[mask]
if np.random.rand() < mutate:
mask = np.random.rand(len(new_chromosome)) < 0.5
new_chromosome[mask] = np.logical_not(new_chromosome[mask])
population.append(new_chromosome)
return np.where(scores[0][1] == 1)[0]
# 运行遗传算法
selected_features = genetic_algorithm(size=100, gensize=X.shape[1], retain=20, random_select=0.05, mutate=0.01)
print(selected_features)
```
在上面的代码中,我们使用SVC作为分类器,定义一个适应度函数来评估每个个体(即特征子集)的好坏程度。遗传算法的主要过程包括初始化种群、评估适应度、选择、交叉和变异。最终输出的是被选择的特征的索引。
阅读全文
相关推荐
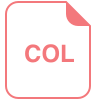
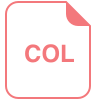
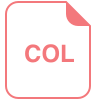
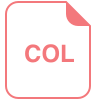
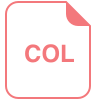
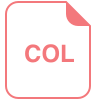
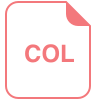
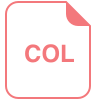
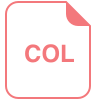
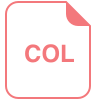
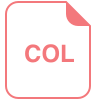
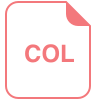
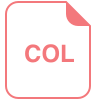
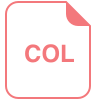
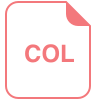