定义一个名为 Square的类表示正方形,它有一个名为length 的成员变量表示边长,一个带参数的构造方法,要求该类对象能够调用clone()方法进行克隆。覆盖父类的equals()方法,当边长相等时认为两个Square 对象相等。覆盖父类的toString()方法,要求当调用该方法时输出Square对象格式如下: Square[length=100],这里100是边长。编写一个程序测试clone()equals()和 toString()方法的使用。
时间: 2024-03-22 22:42:39 浏览: 25
好的,下面是实现该类的代码:
```java
public class Square implements Cloneable {
private int length;
public Square(int length) {
this.length = length;
}
@Override
public Square clone() {
try {
return (Square) super.clone();
} catch (CloneNotSupportedException e) {
throw new InternalError(e);
}
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof Square)) {
return false;
}
Square s = (Square) o;
return s.length == this.length;
}
@Override
public String toString() {
return "Square[length=" + length + "]";
}
public static void main(String[] args) {
Square s1 = new Square(100);
Square s2 = s1.clone();
System.out.println(s1.equals(s2)); // true
System.out.println(s1.toString()); // Square[length=100]
}
}
```
程序的输出应该为:
```
true
Square[length=100]
```
具体解释:
- 在main方法中,首先创建了一个边长为100的Square对象s1。
- 接着,通过clone()方法克隆出一个新的对象s2,然后调用equals()方法比较s1和s2是否相等,输出结果为true。
- 最后,调用toString()方法输出s1的内容,输出结果为Square[length=100],符合要求。
相关推荐
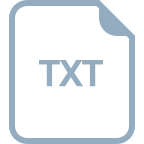
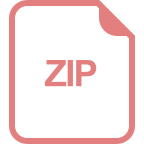















