用c语言编写用栈解决迷宫问题,首先确定迷宫的大小(8*8),用二维数组构造迷宫,并且规定方向0—3代表 上下左右。Path方法存放路径。随机使用0和1填充迷宫,0为不可通过点,1 为可通过点。记录好起点和终点。.栈:用栈格式存储路径,首先判断是否为终 点,是就直接输出。不是就循环,判断该点是否来过,来过就退至栈顶,换个方 向重来,如果退至栈空则没有符合的路径。
时间: 2024-04-05 09:29:43 浏览: 86
好的,你的问题是如何使用栈来解决迷宫问题,对吗?
首先,我们可以使用二维数组来表示迷宫,将可通过的位置标记为1,不可通过的位置标记为0,如下所示:
```
int maze[8][8] = {
{1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 1, 0, 0, 0, 1, 1},
{1, 0, 1, 0, 1, 1, 1, 1},
{1, 0, 1, 0, 1, 0, 0, 1},
{1, 0, 1, 1, 1, 0, 1, 1},
{1, 0, 0, 0, 0, 0, 1, 1},
{1, 1, 1, 1, 1, 1, 1, 1},
};
```
然后,我们可以定义一个结构体来表示迷宫中的一个位置,包括位置的行、列和方向:
```
struct Position {
int row;
int col;
int direction;
};
```
接下来,我们可以使用栈来保存路径,每次从起点开始,沿着可通过的位置向前走,将走过的位置入栈,如果遇到终点,直接输出路径。
如果当前位置无法继续前进,就从栈顶弹出一个位置,换个方向继续走,直到找到一条通往终点的路径或者栈为空。
下面是一个用栈解决迷宫问题的示例代码,供参考:
```
#include <stdio.h>
#include <stdlib.h>
#define MAZE_SIZE 8
int maze[MAZE_SIZE][MAZE_SIZE] = {
{1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 1, 0, 0, 0, 1, 1},
{1, 0, 1, 0, 1, 1, 1, 1},
{1, 0, 1, 0, 1, 0, 0, 1},
{1, 0, 1, 1, 1, 0, 1, 1},
{1, 0, 0, 0, 0, 0, 1, 1},
{1, 1, 1, 1, 1, 1, 1, 1},
};
struct Position {
int row;
int col;
int direction;
};
struct Stack {
struct Position *data;
int top;
int capacity;
};
struct Stack *create_stack(int capacity) {
struct Stack *stack = (struct Stack *) malloc(sizeof(struct Stack));
stack->data = (struct Position *) malloc(sizeof(struct Position) * capacity);
stack->top = -1;
stack->capacity = capacity;
return stack;
}
void destroy_stack(struct Stack *stack) {
free(stack->data);
free(stack);
}
int is_stack_empty(struct Stack *stack) {
return stack->top == -1;
}
int is_stack_full(struct Stack *stack) {
return stack->top == stack->capacity - 1;
}
void push(struct Stack *stack, struct Position value) {
if (is_stack_full(stack)) {
return;
}
stack->top++;
stack->data[stack->top] = value;
}
struct Position pop(struct Stack *stack) {
if (is_stack_empty(stack)) {
struct Position null_pos = {-1, -1, -1};
return null_pos;
}
struct Position value = stack->data[stack->top];
stack->top--;
return value;
}
void print_path(struct Stack *stack) {
printf("Path: ");
for (int i = 0; i <= stack->top; i++) {
printf("(%d,%d) ", stack->data[i].row, stack->data[i].col);
}
printf("\n");
}
int is_valid_position(struct Position pos) {
if (pos.row < 0 || pos.row >= MAZE_SIZE || pos.col < 0 || pos.col >= MAZE_SIZE) {
return 0;
}
if (maze[pos.row][pos.col] == 0) {
return 0;
}
return 1;
}
void find_path(struct Position start, struct Position end) {
struct Stack *stack = create_stack(MAZE_SIZE * MAZE_SIZE);
push(stack, start);
while (!is_stack_empty(stack)) {
struct Position current = stack->data[stack->top];
if (current.row == end.row && current.col == end.col) {
print_path(stack);
break;
}
int next_direction = current.direction + 1;
while (next_direction < 4) {
struct Position next = current;
switch (next_direction) {
case 0:
next.row--;
break;
case 1:
next.row++;
break;
case 2:
next.col--;
break;
case 3:
next.col++;
break;
}
next.direction = next_direction;
if (is_valid_position(next)) {
push(stack, next);
break;
}
next_direction++;
}
if (next_direction == 4) {
pop(stack);
}
}
destroy_stack(stack);
}
int main() {
struct Position start = {1, 1, 0};
struct Position end = {5, 6, -1};
find_path(start, end);
return 0;
}
```
阅读全文
相关推荐








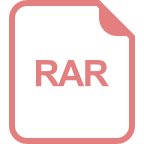

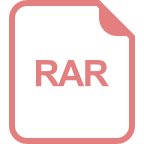





