C++图书馆管理系统利用Easyx代码
时间: 2024-05-04 14:19:16 浏览: 11
以下是一个简单的C++图书馆管理系统的Easyx代码,包括添加图书、删除图书、查找图书和显示所有图书等基本功能。
```c++
#include <graphics.h>
#include <conio.h>
#include <iostream>
#include <fstream>
#include <cstring>
#include <cstdio>
using namespace std;
#define MAX 1000
struct book {
char bookname[20];
char author[10];
char id[10];
char publish[20];
char price[10];
} book[MAX];
int n;
void menu();
void add();
void search();
void show();
void del();
void save();
void read();
int main()
{
initgraph(640, 480);
setbkcolor(WHITE);
cleardevice();
settextcolor(RED);
settextstyle(30, 0, "黑体");
outtextxy(220, 50, "图书管理系统");
read();
menu();
save();
closegraph();
return 0;
}
void menu()
{
cleardevice();
settextcolor(BLUE);
settextstyle(20, 0, "楷体");
outtextxy(50, 80, "1.添加图书");
outtextxy(50, 130, "2.查找图书");
outtextxy(50, 180, "3.删除图书");
outtextxy(50, 230, "4.显示所有图书");
outtextxy(50, 280, "5.退出系统");
int i;
char ch = getch();
switch(ch) {
case '1': add(); break;
case '2': search(); break;
case '3': del(); break;
case '4': show(); break;
case '5': break;
default: menu(); break;
}
}
void add()
{
cleardevice();
settextcolor(BLUE);
settextstyle(20, 0, "楷体");
outtextxy(50, 80, "请输入图书信息:");
outtextxy(50, 130, "书名:");
outtextxy(50, 180, "作者:");
outtextxy(50, 230, "编号:");
outtextxy(50, 280, "出版社:");
outtextxy(50, 330, "价格:");
settextcolor(RED);
settextstyle(20, 0, "宋体");
char ch = getch();
int i;
for(i = 0; i < MAX; i++) {
if(book[i].id[0] == '\0') {
break;
}
}
switch(ch) {
case '1':
cin >> book[i].bookname;
break;
case '2':
cin >> book[i].author;
break;
case '3':
cin >> book[i].id;
break;
case '4':
cin >> book[i].publish;
break;
case '5':
cin >> book[i].price;
break;
case 13:
n++;
menu();
break;
case 27:
menu();
break;
default:
add();
break;
}
add();
}
void search()
{
cleardevice();
settextcolor(BLUE);
settextstyle(20, 0, "楷体");
outtextxy(50, 80, "请输入要查找的图书编号:");
settextcolor(RED);
settextstyle(20, 0, "宋体");
char ch = getch();
int i;
for(i = 0; i < n; i++) {
if(strcmp(book[i].id, ch) == 0) {
cout << book[i].bookname << " " << book[i].author << " " << book[i].id << " " << book[i].publish << " " << book[i].price << endl;
break;
}
}
if(i == n) {
cout << "未找到该图书,请重新输入!" << endl;
search();
} else {
menu();
}
}
void show()
{
cleardevice();
settextcolor(BLUE);
settextstyle(20, 0, "楷体");
outtextxy(50, 80, "所有图书信息如下:");
settextcolor(RED);
settextstyle(20, 0, "宋体");
for(int i = 0; i < n; i++) {
cout << book[i].bookname << " " << book[i].author << " " << book[i].id << " " << book[i].publish << " " << book[i].price << endl;
}
getch();
menu();
}
void del()
{
cleardevice();
settextcolor(BLUE);
settextstyle(20, 0, "楷体");
outtextxy(50, 80, "请输入要删除的图书编号:");
settextcolor(RED);
settextstyle(20, 0, "宋体");
char ch = getch();
int i;
for(i = 0; i < n; i++) {
if(strcmp(book[i].id, ch) == 0) {
for(int j = i; j < n-1; j++) {
book[j] = book[j+1];
}
n--;
break;
}
}
if(i == n) {
cout << "未找到该图书,请重新输入!" << endl;
del();
} else {
menu();
}
}
void save()
{
ofstream fout("book.txt");
for(int i = 0; i < n; i++) {
fout << book[i].bookname << " " << book[i].author << " " << book[i].id << " " << book[i].publish << " " << book[i].price << endl;
}
fout.close();
}
void read()
{
ifstream fin("book.txt");
while(!fin.eof()) {
fin >> book[n].bookname >> book[n].author >> book[n].id >> book[n].publish >> book[n].price;
n++;
}
fin.close();
}
```
此代码仅供参考,可以根据实际情况进行修改和完善。
相关推荐
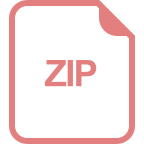
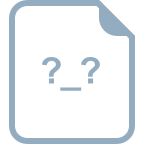














