pytorch 实现lstm注意力
时间: 2023-07-31 22:11:39 浏览: 97
在PyTorch中实现LSTM注意力,可以参考以下代码:
```python
import torch
import torch.nn as nn
class LSTMAttention(nn.Module):
def __init__(self, input_size, hidden_size, num_layers):
super(LSTMAttention, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, 1)
def forward(self, x):
# x的形状为(batch_size, sequence_length, input_size)
outputs, _ = self.lstm(x) # 经过LSTM层得到输出outputs,形状为(batch_size, sequence_length, hidden_size)
scores = self.fc(outputs).squeeze(2) # 将输出经过一个全连接层得到注意力分数,形状为(batch_size, sequence_length)
attention_weights = torch.softmax(scores, dim=1).unsqueeze(2) # 对注意力分数进行softmax得到注意力权重,形状为(batch_size, sequence_length, 1)
weighted_outputs = (outputs * attention_weights).sum(dim=1) # 将加权后的输出求和得到加权输出,形状为(batch_size, hidden_size)
return weighted_outputs
```
这里定义了一个`LSTMAttention`模型,它继承了`nn.Module`类。在模型的`__init__`方法中,初始化了一个LSTM层和一个全连接层,其中LSTM层的输入维度为`input_size`,输出维度为`hidden_size`,层数为`num_layers`。在模型的`forward`方法中,将输入`x`经过LSTM层得到输出`outputs`,然后将`outputs`经过全连接层得到注意力分数`scores`。接着,对注意力分数进行softmax得到注意力权重`attention_weights`,最后将加权后的输出求和得到加权输出`weighted_outputs`。注意,这里`squeeze`和`unsqueeze`函数的作用是调整张量的形状,使得它们能够进行正确的计算。
阅读全文
相关推荐
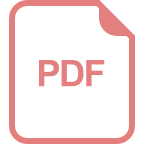

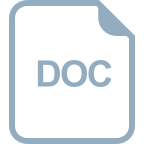
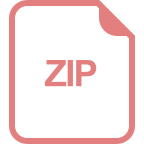
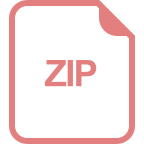
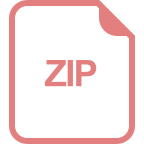
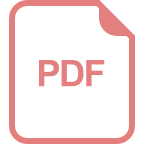
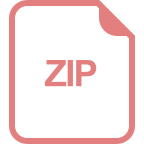
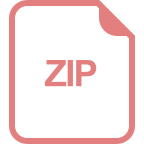
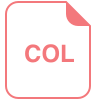




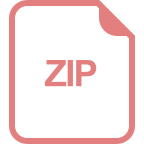
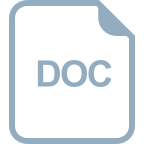
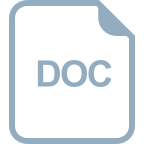