DataFrame对象 data.assign() 函数
时间: 2024-05-12 21:16:37 浏览: 11
DataFrame对象是Pandas库中的一种数据结构,类似于Excel中的表格。它由行和列组成,每一列可以是不同的数据类型(例如整数、浮点数、字符串等)。DataFrame对象可以用于数据清洗、数据分析和数据可视化等多个方面。
data.assign()函数是DataFrame对象的一个方法,用于在现有的DataFrame对象中添加新的列。该方法可以接受一个或多个参数,每个参数都是一个新列的名称和该列的数据。例如,以下代码将在名为df的DataFrame对象中添加一个名为new_column的新列,并将该列的值设置为1:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.assign(new_column=1)
```
此时,df的值将如下所示:
```
A B new_column
0 1 4 1
1 2 5 1
2 3 6 1
```
注意,assign()函数返回一个新的DataFrame对象,因此需要将其赋值给一个变量。如果要在原始DataFrame对象中添加新列,可以使用inplace参数:
```python
df.assign(new_column=1, inplace=True)
```
相关问题
DataFrame 选择列
在Python中,可以使用pandas库中的DataFrame对象来添加列。具体步骤如下:
1. 创建一个DataFrame对象,可以使用pandas库中的read_csv()函数从CSV文件中读取数据,也可以手动创建一个DataFrame对象。
2. 使用DataFrame对象的assign()方法来添加新列,该方法接受一个字典作为参数,字典的键为新列的名称,值为新列的数据。
3. 使用DataFrame对象的insert()方法来插入新列,该方法接受三个参数,第一个参数为新列的位置,第二个参数为新列的名称,第三个参数为新列的数据。
例如,以下代码演示了如何在DataFrame对象中添加新列:
```
import pandas as pd
# 创建一个DataFrame对象
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# 使用assign()方法添加新列
df = df.assign(gender=['F', 'M', 'M', 'M'])
# 使用insert()方法插入新列
df.insert(2, 'salary', [50000, 60000, 70000, 80000])
print(df)
```
输出结果为:
```
name age salary gender
Alice 25 50000 F
1 Bob 30 60000 M
2 Charlie 35 70000 M
3 David 40 80000 M
```
python dataframe添加列
在Python中,可以使用pandas库中的DataFrame对象来添加列。具体步骤如下:
1. 创建一个DataFrame对象,可以使用pandas库中的read_csv()函数从CSV文件中读取数据,也可以手动创建一个DataFrame对象。
2. 使用DataFrame对象的assign()方法来添加新列,该方法接受一个字典作为参数,字典的键为新列的名称,值为新列的数据。
3. 使用DataFrame对象的insert()方法来插入新列,该方法接受三个参数,第一个参数为新列的位置,第二个参数为新列的名称,第三个参数为新列的数据。
例如,以下代码演示了如何在DataFrame对象中添加新列:
```
import pandas as pd
# 创建一个DataFrame对象
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
# 使用assign()方法添加新列
df = df.assign(gender=['F', 'M', 'M', 'M'])
# 使用insert()方法插入新列
df.insert(2, 'salary', [50000, 60000, 70000, 80000])
print(df)
```
输出结果为:
```
name age salary gender
Alice 25 50000 F
1 Bob 30 60000 M
2 Charlie 35 70000 M
3 David 40 80000 M
```
相关推荐
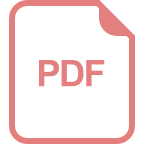
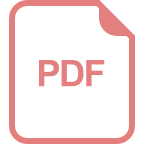
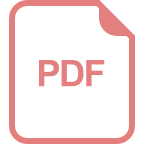












