用cpp语言制作一个程序,要求写出完整的代码,该程序要求实现散列表中的查找和插入功能.
时间: 2023-02-05 12:05:46 浏览: 72
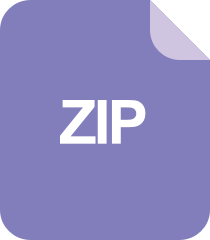
Favorite.cpp:这是一个开源程序,要求您喜欢的编程语言。随时提出一些更改!
我们可以先定义一个结构体来表示散列表中的每一个节点,其中包含一个键值和一个值,然后再定义一个结构体来表示散列表本身,其中包含一个桶的数组和一个表示散列表大小的变量。
下面是实现查找和插入功能的代码:
```cpp
#include <iostream>
#include <unordered_map>
using namespace std;
struct Node {
int key;
int value;
Node *next;
Node(int k, int v) : key(k), value(v), next(NULL) {}
};
struct HashTable {
int size;
Node **bucket;
HashTable(int s) : size(s) {
bucket = new Node*[size];
for (int i = 0; i < size; i++) {
bucket[i] = NULL;
}
}
int hash(int key) {
return key % size;
}
void insert(int key, int value) {
int index = hash(key);
Node *node = new Node(key, value);
node->next = bucket[index];
bucket[index] = node;
}
Node *search(int key) {
int index = hash(key);
Node *node = bucket[index];
while (node != NULL) {
if (node->key == key) {
return node;
}
node = node->next;
}
return NULL;
}
};
int main() {
HashTable ht(10);
ht.insert(1, 10);
ht.insert(2, 20);
ht.insert(3, 30);
Node *node = ht.search(2);
if (node == NULL) {
cout << "Key not found" << endl;
} else {
cout << "Key found: " << node->value << endl;
}
return 0;
}
```
请注意,上面的代码使用的是链地址法来解决散列冲突的问题,但也可以使用其他的解决方案,例如开放地址法。
阅读全文
相关推荐
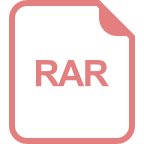















